怎么将图片中的水印识别后提取出来 代码
时间: 2024-09-25 08:05:51 浏览: 18
将图片中的水印识别并提取出来通常涉及图像处理和计算机视觉技术。这里提供一个简化的步骤概述,以及一个Python示例,使用OpenCV库,但请注意,这需要一定的编程基础和可能需要深度学习模型如神经网络来提高精度。
1. **安装必要的库**:
- 安装`opencv-python` 和 `numpy`: `pip install opencv-python numpy`
2. **基本流程**:
- 图像读取: 使用`cv2.imread()`函数加载图片。
- 检测水印区域: 这可能涉及到低级的边缘检测、色彩分析(比如对比度或相似性),或更高级的特征匹配技术。
- 提取像素: 根据检测到的边界或特征定位,选择特定区域提取像素。
- 可能还需要预处理(例如灰度化、二值化)以便于后续操作。
3. **简单Python代码示例** (仅作参考,实际效果取决于水印的复杂性和可用资源):
```python
import cv2
import numpy as np
def extract_watermark(image_path):
# 1. 读取图片
img = cv2.imread(image_path)
# 2. 转换为灰度图
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 3. 进行边缘检测,假设水印是一个较明显的边缘(可以尝试其他算法)
edges = cv2.Canny(gray_img, threshold1=50, threshold2=150)
# 4. 找出边缘轮廓
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 5. 遍历轮廓,选择可能的水印区域
watermark_region = None
for contour in contours:
area = cv2.contourArea(contour)
if area > 0.1 * img.shape[0] * img.shape[1]: # 简单过滤较小面积的区域
x, y, w, h = cv2.boundingRect(contour)
watermark_region = img[y:y+h, x:x+w]
return watermark_region
# 使用方法
watermarked_image = extract_watermark("path_to_your_image.jpg")
if watermark_region is not None:
cv2.imwrite("extracted_watermark.jpg", watermark_region)
else:
print("Watermark not found.")
```
相关推荐
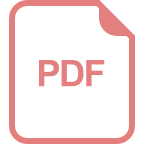
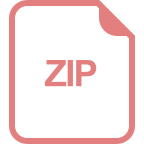
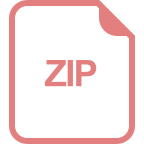


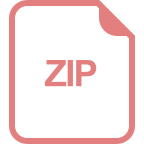
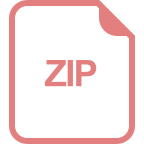
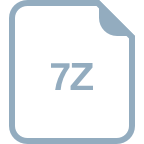
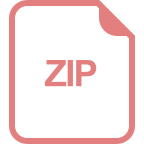
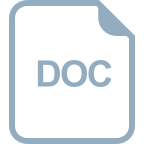
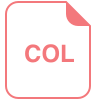



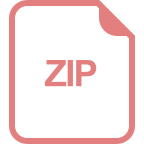
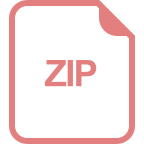
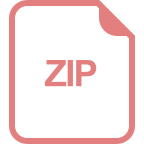