编写程序,建立整型向量容器,从键盘上输人若干正整数,添加在向量容器尾部, 输出向量容器内所有元素,再将向量容器内所有素数拷贝至整型双端队列容器内,再输出 整型双端队列容器内所有元素,要求使用lambda函数。
时间: 2024-03-21 16:40:22 浏览: 69
好的,我会尽力回答你的问题。下面是程序的实现:
```c++
#include <iostream>
#include <vector>
#include <deque>
#include <algorithm>
using namespace std;
int main() {
vector<int> vec; // 整型向量容器
int num;
cout << "请输入若干正整数,以0结束:" << endl;
while (cin >> num && num != 0) {
vec.push_back(num);
}
cout << "整型向量容器内所有元素:" << endl;
for (auto it = vec.begin(); it != vec.end(); ++it) {
cout << *it << " ";
}
cout << endl;
deque<int> dq; // 整型双端队列容器
auto is_prime = [](int n) {
if (n < 2) return false;
for (int i = 2; i * i <= n; ++i) {
if (n % i == 0) return false;
}
return true;
};
copy_if(vec.begin(), vec.end(), back_inserter(dq), is_prime);
cout << "整型双端队列容器内所有素数元素:" << endl;
for (auto it = dq.begin(); it != dq.end(); ++it) {
cout << *it << " ";
}
cout << endl;
return 0;
}
```
程序中使用了 `vector` 容器来实现整型向量,并通过 `push_back` 函数将输入的正整数添加到向量的尾部。然后使用 lambda 函数 `is_prime` 判断素数,并使用 `copy_if` 函数将向量中的素数拷贝到双端队列容器中,其中的判断素数的 lambda 函数为 `is_prime`。最后输出向量容器和双端队列容器中的元素。
注意:上述程序中,为了方便起见,我们将输入的若干正整数以 0 结束。如果需要改变这个结束标志,可以在程序中相应地修改。
阅读全文
相关推荐
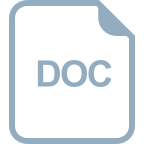
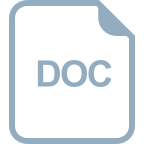
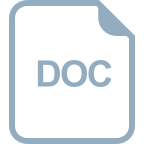



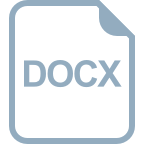
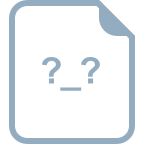
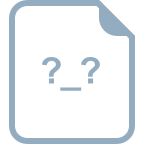
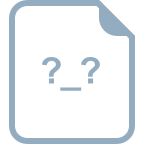
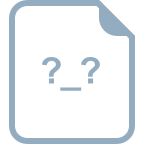
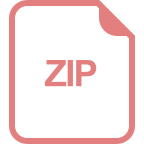
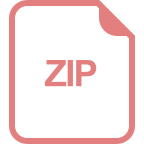
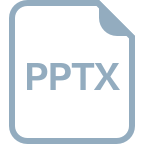
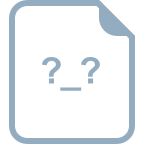


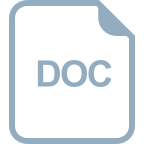