PIL.Image转np
时间: 2023-12-04 10:02:55 浏览: 150
将PIL.Image对象转换为NumPy数组可以使用NumPy库中的asarray()函数。以下是将PIL.Image对象转换为NumPy数组的示例代码:
```python
from PIL import Image
import numpy as np
# 打开图像
image = Image.open("image.jpg")
# 将图像转换为NumPy数组
image_array = np.asarray(image)
# 打印数组的形状和数据类型
print("数组形状:", image_array.shape)
print("数据类型:", image_array.dtype)
```
在上面的代码中,"image.jpg"是你要转换的图像文件的路径。使用np.asarray()函数将PIL.Image对象转换为NumPy数组,并将结果存储在image_array变量中。你可以通过打印数组的形状和数据类型来验证转换是否成功。
请确保你已经安装了NumPy库,它提供了对NumPy数组进行操作和处理的各种功能。
相关问题
class Image2Array(object): """ transfer PIL.Image to Numpy array and transpose dimensions from 'dhwc' to 'dchw'. Args: transpose: whether to transpose or not, default True, False for slowfast. """ def __init__(self, transpose=True): self.transpose = transpose def __call__(self, results): """ Performs Image to NumpyArray operations. Args: imgs: List where each item is a PIL.Image. For example, [PIL.Image0, PIL.Image1, PIL.Image2, ...] return: np_imgs: Numpy array. """ imgs = results['imgs'] np_imgs = (np.stack(imgs)).astype('float32') if self.transpose: np_imgs = np_imgs.transpose(0, 3, 1, 2) # tchw results['imgs'] = np_imgs return results
这是一个Python类,用于将PIL.Image对象转换为Numpy数组,并且可以选择是否转置数组的维度。它可以作为数据处理管道中的一个步骤,例如在图像分类或物体检测任务中。下面是一个简单的示例代码,演示如何将图像文件转换为Numpy数组,并应用Image2Array类:
```python
from PIL import Image
import numpy as np
class Image2Array(object):
"""
transfer PIL.Image to Numpy array and transpose dimensions from 'dhwc' to 'dchw'.
Args:
transpose: whether to transpose or not, default True, False for slowfast.
"""
def __init__(self, transpose=True):
self.transpose = transpose
def __call__(self, results):
"""
Performs Image to NumpyArray operations.
Args:
imgs: List where each item is a PIL.Image.
For example, [PIL.Image0, PIL.Image1, PIL.Image2, ...]
return:
np_imgs: Numpy array.
"""
imgs = results['imgs']
np_imgs = (np.stack(imgs)).astype('float32')
if self.transpose:
np_imgs = np_imgs.transpose(0, 3, 1, 2) # tchw
results['imgs'] = np_imgs
return results
# 读取图像文件
img = Image.open("image.jpg")
# 应用Image2Array类将图像转换为Numpy数组
image2array = Image2Array()
np_img = image2array({'imgs': [img]})['imgs'][0]
# 输出数组形状
print(np_img.shape)
```
在上面的代码中,我们首先使用PIL库打开一个图像文件,然后使用Image2Array类将其转换为Numpy数组。最后,我们输出数组的形状。注意,我们将图像文件转换为单个PIL.Image对象,并将其放入一个列表中,因为Image2Array类预期的输入是一个图像列表。
PIL.Image转换成OpenCV格式
你可以使用以下代码将PIL.Image转换为OpenCV格式:
```python
import cv2
from PIL import Image
pil_image = Image.open("example.jpg") # 读取PIL.Image
opencv_image = cv2.cvtColor(np.array(pil_image), cv2.COLOR_RGB2BGR) # 转换为OpenCV格式
```
需要注意的是,需要先安装Pillow和OpenCV库。
阅读全文
相关推荐
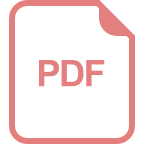
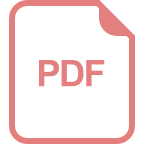


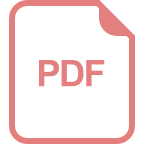
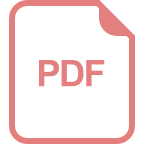
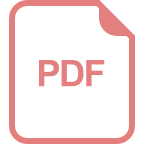







