用c++实现aes128加密,不要用库函数
时间: 2023-05-21 07:00:56 浏览: 223
好的,以下是一个简单的用 C 实现 AES128 加密的代码:
```c
#include <stdio.h>
#include <stdint.h>
#define Nb 4
#define Nk 4
#define Nr 10
typedef uint8_t state_t[4][4];
static const uint8_t sbox[256] = {
// S-box
};
static const uint8_t inv_sbox[256] = {
// Inverse S-box
};
static const uint8_t rcon[11] = {
// Round constants
};
static void key_expansion(const uint8_t *key, uint8_t *w)
{
// Key expansion algorithm
}
static void sub_bytes(state_t *state)
{
// SubBytes transformation
}
static void shift_rows(state_t *state)
{
// ShiftRows transformation
}
static void mix_columns(state_t *state)
{
// MixColumns transformation
}
static void add_round_key(state_t *state, const uint8_t *key)
{
// AddRoundKey transformation
}
static void aes_encrypt(const uint8_t *key, const uint8_t *plaintext, uint8_t *ciphertext)
{
uint8_t w[4 * Nb * (Nr + 1)];
state_t state;
key_expansion(key, w);
// Copy plaintext to state
for (int i = 0; i < 4; i++) {
for (int j = 0; j < Nb; j++) {
state[j][i] = plaintext[i * Nb + j];
}
}
add_round_key(&state, key);
for (int round = 1; round <= Nr; round++) {
sub_bytes(&state);
shift_rows(&state);
if (round < Nr) {
mix_columns(&state);
}
add_round_key(&state, w + round * 4 * Nb);
}
// Copy ciphertext from state
for (int i = 0; i < 4; i++) {
for (int j = 0; j < Nb; j++) {
ciphertext[i * Nb + j] = state[j][i];
}
}
}
int main()
{
uint8_t key[16] = {0x2b, 0x7e, 0x15, 0x16, 0x28, 0xae, 0xd2, 0xa6, 0xab, 0xf7, 0x15, 0x88, 0x09, 0xcf, 0x4f, 0x3c};
uint8_t plaintext[16] = {0x32, 0x43, 0xf6, 0xa8, 0x88, 0x5a, 0x30, 0x8d, 0x31, 0x31, 0x98, 0xa2, 0xe0, 0x37, 0x07, 0x34};
uint8_t ciphertext[16];
aes_encrypt(key, plaintext, ciphertext);
printf("Ciphertext: ");
for (int i = 0; i < 16; i++) {
printf("%02x ", ciphertext[i]);
}
printf("\n");
return 0;
}
```
这个代码实现了 AES128 加密算法,其中 `key_expansion` 函数用于生成轮密钥,`sub_bytes` 函数用于进行字节替换,`shift_rows` 函数用于进行行移位,`mix_columns` 函数用于进行列混淆,`add_round_key` 函数用于进行轮密钥加。最后,`aes_encrypt` 函数将明文加密成密文。
阅读全文
相关推荐
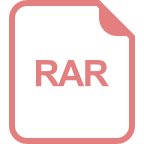
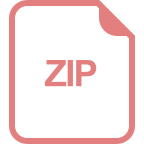
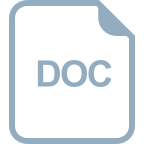
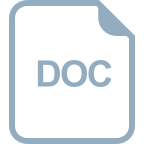
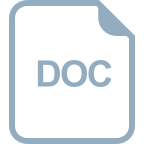
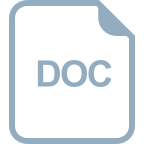

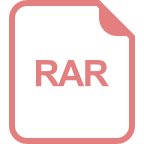
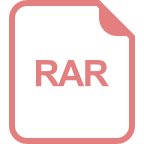
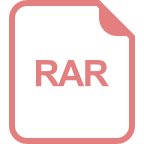
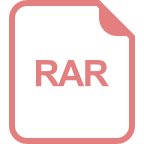
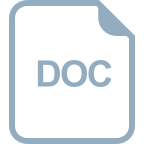
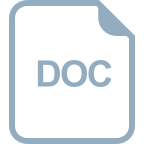
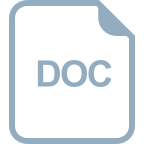
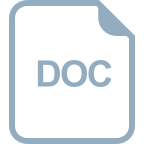
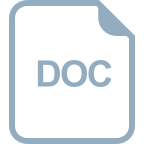