C++如何读取文件数据(读取从指定的连在一起的字符开始,到指定的连在一起的字符结束)
时间: 2024-10-10 14:15:27 浏览: 60
在C++中,你可以使用`ifstream`类来读取文件数据。如果你想要从文件中的特定位置开始读取,直到遇到某个特定的终止字符为止,你需要首先定位到那个起始位置,然后继续读取直到遇到终止字符。下面是一个简单的示例:
```cpp
#include <fstream>
#include <string>
std::string readUntilTermination(const std::string& filename, size_t startOffset, char terminator) {
std::ifstream inputFile(filename, std::ios::binary); // 使用二进制模式以处理非文本字符
if (!inputFile) {
throw std::runtime_error("Failed to open file.");
}
inputFile.seekg(startOffset, std::ios::beg); // 移动到起始位置
std::string content;
char currentChar;
while (inputFile.get(currentChar)) {
if (currentChar == terminator) {
break; // 找到了终止字符,跳出循环
}
content.push_back(currentChar); // 将字符追加到字符串中
}
inputFile.close(); // 关闭文件
return content; // 返回包含读取部分的字符串
}
int main() {
try {
std::string data = readUntilTermination("example.txt", 1000, '\n'); // 读取直到换行符
if (!data.empty()) {
std::cout << "Data from specified location until termination character: " << data << std::endl;
} else {
std::cout << "No data found or reached end of file before termination character." << std::endl;
}
} catch (const std::exception& e) {
std::cerr << "Error reading file: " << e.what() << std::endl;
}
return 0;
}
```
这个函数从指定的起始位置开始读取,直到遇到指定的终止字符(如'\n'代表换行)。注意,如果到达了文件末尾还没找到终止字符,或者打开文件失败,程序会抛出异常。
阅读全文
相关推荐
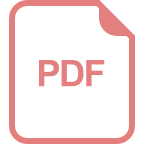
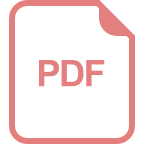
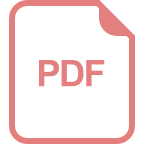
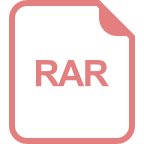
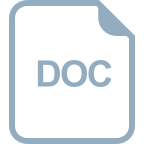
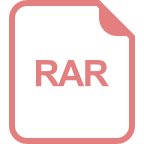
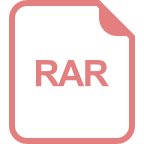
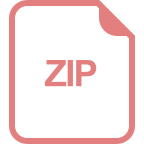
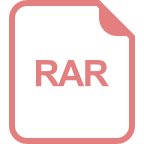
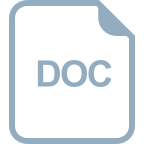
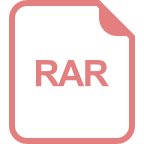
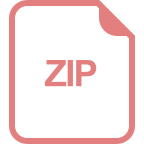
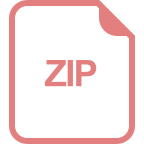
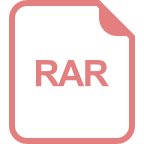
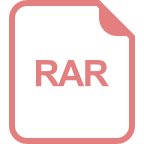
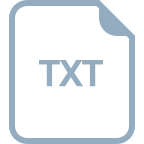
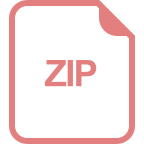
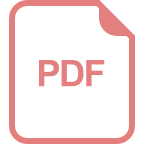
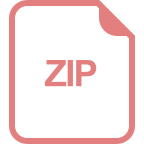