用WinAPI实现一个图片查看器:一个打开按钮,点击后打开一个打开文件对话框,打开选中的png文件显示在屏幕上,并可以用鼠标滚轮调整大小
时间: 2024-04-12 08:31:09 浏览: 21
你可以使用WinAPI来实现一个图片查看器,以下是一个基本的示例代码:
```cpp
#include <windows.h>
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) {
// 创建窗口类
WNDCLASS wc = { 0 };
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.lpszClassName = "ImageViewer";
// 注册窗口类
RegisterClass(&wc);
// 创建窗口
HWND hwnd = CreateWindow("ImageViewer", "Image Viewer", WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT, 800, 600, NULL, NULL, hInstance, NULL);
// 显示窗口
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam) {
static HBITMAP hBitmap = NULL;
switch (msg) {
case WM_CREATE: {
// 创建打开按钮
CreateWindow("BUTTON", "Open", WS_VISIBLE | WS_CHILD, 10, 10, 80, 30, hwnd, (HMENU)1, NULL, NULL);
break;
}
case WM_COMMAND: {
if (LOWORD(wParam) == 1) {
// 打开文件对话框
char szFile[MAX_PATH] = { 0 };
OPENFILENAME ofn = { 0 };
ofn.lStructSize = sizeof(ofn);
ofn.hwndOwner = hwnd;
ofn.lpstrFile = szFile;
ofn.nMaxFile = sizeof(szFile);
ofn.lpstrFilter = "PNG Files\0*.png\0All Files\0*.*\0";
ofn.nFilterIndex = 1;
ofn.Flags = OFN_PATHMUSTEXIST | OFN_FILEMUSTEXIST;
if (GetOpenFileName(&ofn)) {
// 加载图片
hBitmap = (HBITMAP)LoadImage(NULL, ofn.lpstrFile, IMAGE_BITMAP, 0, 0, LR_LOADFROMFILE);
// 刷新窗口
InvalidateRect(hwnd, NULL, TRUE);
}
}
break;
}
case WM_PAINT: {
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
// 绘制图片
if (hBitmap) {
HDC hdcMem = CreateCompatibleDC(hdc);
HBITMAP hOldBitmap = (HBITMAP)SelectObject(hdcMem, hBitmap);
BITMAP bmp;
GetObject(hBitmap, sizeof(BITMAP), &bmp);
int width = bmp.bmWidth;
int height = bmp.bmHeight;
// 调整图片大小以适应窗口
RECT clientRect;
GetClientRect(hwnd, &clientRect);
float aspectRatio = (float)width / height;
int newWidth = clientRect.right - clientRect.left;
int newHeight = (int)(newWidth / aspectRatio);
if (newHeight > clientRect.bottom - clientRect.top) {
newHeight = clientRect.bottom - clientRect.top;
newWidth = (int)(newHeight * aspectRatio);
}
// 居中绘制
int x = (clientRect.right - clientRect.left - newWidth) / 2;
int y = (clientRect.bottom - clientRect.top - newHeight) / 2;
StretchBlt(hdc, x, y, newWidth, newHeight, hdcMem, 0, 0, width, height, SRCCOPY);
SelectObject(hdcMem, hOldBitmap);
DeleteDC(hdcMem);
}
EndPaint(hwnd, &ps);
break;
}
case WM_MOUSEWHEEL: {
// 使用鼠标滚轮调整图片大小
if (hBitmap) {
int delta = GET_WHEEL_DELTA_WPARAM(wParam);
float scaleFactor = 1.1f;
if (delta > 0) {
// 向上滚动,放大图片
int newWidth = (int)(GetWidth(hBitmap) * scaleFactor);
int newHeight = (int)(GetHeight(hBitmap) * scaleFactor);
hBitmap = (HBITMAP)CopyImage(hBitmap, IMAGE_BITMAP, newWidth, newHeight, LR_COPYRETURNORG);
} else {
// 向下滚动,缩小图片
int newWidth = (int)(GetWidth(hBitmap) / scaleFactor);
int newHeight = (int)(GetHeight(hBitmap) / scaleFactor);
hBitmap = (HBITMAP)CopyImage(hBitmap, IMAGE_BITMAP, newWidth, newHeight, LR_COPYRETURNORG);
}
// 刷新窗口
InvalidateRect(hwnd, NULL, TRUE);
}
break;
}
case WM_DESTROY: {
// 释放资源
if (hBitmap) {
DeleteObject(hBitmap);
}
PostQuitMessage(0);
break;
}
default: {
return DefWindowProc(hwnd, msg, wParam, lParam);
}
}
return 0;
}
```
这段代码创建了一个简单的窗口应用程序,包含一个"Open"按钮和一个用于显示图片的窗口。点击"Open"按钮会打开一个文件对话框,选择一个PNG文件后,该文件将会显示在窗口中。你可以使用鼠标滚轮来调整图片的大小。注意,这段代码并没有进行错误处理和内存管理,你可以根据自己的需要进行相应的改进。
相关推荐
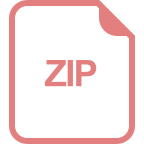
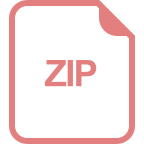
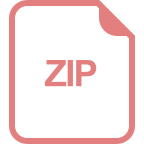













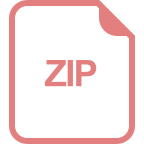