C++打开指定文件路径的文件使用winapi,并显示内容在窗口中
时间: 2024-05-07 22:21:18 浏览: 145
下面是一个使用WinAPI打开指定文件路径的文件,并将其内容显示在窗口中的C++示例代码:
```cpp
#include <windows.h>
#include <string>
#include <fstream>
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
// 创建窗口
const std::string className = "MyWindowClass";
const std::string windowName = "My Window";
const int width = 800;
const int height = 600;
WNDCLASSEX wcex = { 0 };
wcex.cbSize = sizeof(WNDCLASSEX);
wcex.style = CS_HREDRAW | CS_VREDRAW;
wcex.lpfnWndProc = WndProc;
wcex.hInstance = hInstance;
wcex.hCursor = LoadCursor(NULL, IDC_ARROW);
wcex.lpszClassName = className.c_str();
RegisterClassEx(&wcex);
HWND hwnd = CreateWindow(className.c_str(), windowName.c_str(), WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT, width, height, NULL, NULL, hInstance, NULL);
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// 打开文件并显示内容
const std::string filePath = "C:\\example.txt";
std::ifstream fileStream(filePath);
if (!fileStream.is_open())
{
MessageBox(hwnd, "Failed to open file!", "Error", MB_ICONERROR | MB_OK);
return 0;
}
std::string fileContent((std::istreambuf_iterator<char>(fileStream)), std::istreambuf_iterator<char>());
fileStream.close();
SetWindowText(hwnd, fileContent.c_str());
// 消息循环
MSG msg = { 0 };
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return (int)msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
switch (message)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, message, wParam, lParam);
}
return 0;
}
```
这个示例程序创建了一个窗口,并在窗口中打开了一个指定路径的文件,并将其内容显示在窗口标题栏中。需要注意的是,这里使用了std::ifstream和std::string来读取文件内容,并使用了SetWindowText函数来设置窗口标题栏的文本。你可以根据自己的需求进行修改。
阅读全文
相关推荐
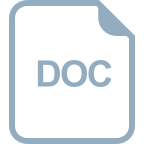
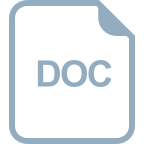
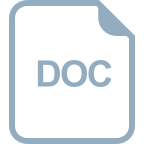
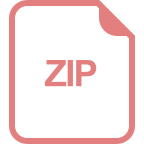
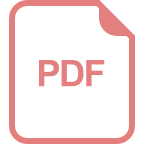
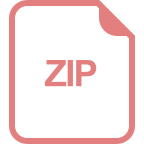
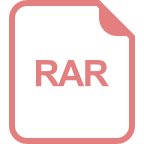
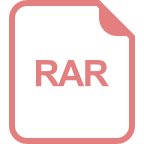
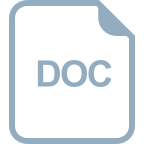









