c++ 根据图片生成异形窗口并在生成的窗口上画图 使用gdi+ 不使用qt 详细代码
时间: 2024-03-05 19:49:52 浏览: 18
好的,我可以为您提供一个基于 C++ 和 GDI+ 的示例代码,用于创建异形窗口并在窗口上绘制图像。下面是代码:
```c++
#include <windows.h>
#include <gdiplus.h>
#pragma comment (lib,"Gdiplus.lib")
// 窗口类名
const wchar_t* WindowClassName = L"MyWindowClass";
// 要绘制的图片路径
const wchar_t* ImageFilePath = L"C:\\image.bmp";
// 窗口句柄
HWND hWnd;
// GDI+ 全局变量
Gdiplus::GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
// 窗口回调函数声明
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
// 初始化 GDI+
Gdiplus::GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
// 注册窗口类
WNDCLASSEX wc;
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = CS_HREDRAW | CS_VREDRAW;
wc.lpfnWndProc = WndProc;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = hInstance;
wc.hIcon = NULL;
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszMenuName = NULL;
wc.lpszClassName = WindowClassName;
wc.hIconSm = NULL;
RegisterClassEx(&wc);
// 创建窗口
hWnd = CreateWindowEx(
WS_EX_LAYERED | WS_EX_TRANSPARENT | WS_EX_TOPMOST,
WindowClassName, L"My Window",
WS_POPUPWINDOW | WS_VISIBLE,
100, 100, 640, 480,
NULL, NULL, hInstance, NULL);
// 设置窗口为异形窗口
HRGN hRgn = CreateEllipticRgn(0, 0, 640, 480);
SetWindowRgn(hWnd, hRgn, TRUE);
// 加载图片
Gdiplus::Bitmap bitmap(ImageFilePath);
// 绘制图片到窗口
HDC hdc = GetDC(hWnd);
Gdiplus::Graphics graphics(hdc);
graphics.DrawImage(&bitmap, 0, 0, 640, 480);
ReleaseDC(hWnd, hdc);
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
// 释放 GDI+
Gdiplus::GdiplusShutdown(gdiplusToken);
return (int)msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
switch (message)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hWnd, &ps);
EndPaint(hWnd, &ps);
}
break;
default:
return DefWindowProc(hwnd, message, wParam, lParam);
}
return 0;
}
```
上面的代码中,我们首先初始化了 GDI+,然后注册了一个窗口类,并创建了一个异形窗口。我们使用 `CreateEllipticRgn` 函数创建一个椭圆形区域作为窗口的形状,然后使用 `SetWindowRgn` 函数设置窗口的形状。接下来,我们加载了一张图片,并使用 GDI+ 绘制到窗口上。最后,在消息循环中,我们处理了窗口的关闭消息,并在窗口绘制时处理了 `WM_PAINT` 消息。
需要注意的是,由于我们创建的是异形窗口,因此窗口的背景应该是透明的。我们通过设置窗口的扩展样式 `WS_EX_LAYERED` 和 `WS_EX_TRANSPARENT` 来实现窗口的透明效果。另外,我们还将窗口的扩展样式设置为 `WS_EX_TOPMOST`,使窗口置于所有其他窗口之上。
希望这个示例代码能够对您有所帮助。
相关推荐
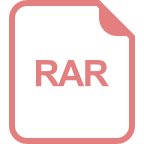
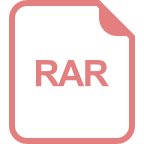














