c++ 生成一个窗口并把base64图像展示出来 不使用qt 使用GDI+
时间: 2024-01-07 22:06:14 浏览: 184
好的,以下是一个使用 GDI+ 在 C++ 中生成窗口并展示 base64 图像的示例代码:
```
#include <windows.h>
#include <gdiplus.h>
#include <string>
#include <iostream>
#pragma comment (lib,"Gdiplus.lib")
using namespace Gdiplus;
using namespace std;
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
PSTR szCmdLine, int iCmdShow)
{
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
// Initialize GDI+
GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
// Register the main window class
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = TEXT("Base64ImageDisplay");
if (!RegisterClass(&wndclass))
{
MessageBox(NULL, TEXT("This program requires Windows NT!"), TEXT("Error"), MB_ICONERROR);
return 0;
}
// Create the main window
hwnd = CreateWindow(TEXT("Base64ImageDisplay"), TEXT("Base64 Image Display"),
WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT,
640, 480, NULL, NULL, hInstance, NULL);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
// Enter the message loop
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
// Shutdown GDI+
GdiplusShutdown(gdiplusToken);
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
PAINTSTRUCT ps;
HDC hdc;
WCHAR* base64Image = L"iVBORw0KGgoAAAANSUhEUgAAABAAAAAQEAYAAABPYyMiAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAAMSURBVDhPYxgF/mYAAICDAv6iURfAAAAAElFTkSuQmCC";
switch (message)
{
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
Graphics graphics(hdc);
// Decode base64 image
ULONG size = 0;
BYTE* bytes = NULL;
Base64Decode((const char*)base64Image, &bytes, &size);
// Load image from memory
HGLOBAL hMem = GlobalAlloc(GMEM_MOVEABLE, size);
LPVOID pMem = GlobalLock(hMem);
memcpy(pMem, bytes, size);
IStream* pStream = NULL;
CreateStreamOnHGlobal(hMem, FALSE, &pStream);
Image image(pStream);
// Draw image
graphics.DrawImage(&image, 0, 0, 640, 480);
// Cleanup
GlobalUnlock(hMem);
GlobalFree(hMem);
delete[] bytes;
EndPaint(hwnd, &ps);
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, message, wParam, lParam);
}
return 0;
}
```
该代码会创建一个名为 "Base64ImageDisplay" 的窗口,并在其中展示一个 base64 编码的图片。你可以将 `base64Image` 替换为你自己的 base64 图片字符串。
注意:此处使用了 GDI+ 库和 base64 解码函数 `Base64Decode()`,需要在编译时链接 GDI+ 库并包含 base64 解码函数的实现。
阅读全文
相关推荐















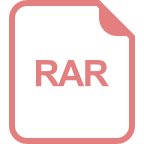


