输入年份和月份,判断输出该年该月有多少天(注意有闰年)。 闰年(Leap year)的条件是符合下面两条件之一: (1)年份能被 4 整除,但不能被 100 整除; (2)年份能被 400 整除。
时间: 2024-10-21 11:13:20 浏览: 16
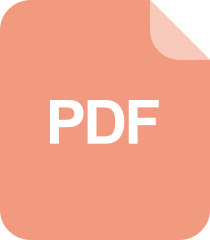
Python编程实现输入某年某月某日计算出这一天是该年第几天的方法
要编写一个程序来计算给定年份和月份的天数,你需要考虑闰年的规则。以下是一个简单的C语言函数,用于根据输入的年份和月份返回该月的天数:
```c
#include <stdio.h>
int is_leap_year(int year) {
if (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) {
return 1; // 是闰年
} else {
return 0; // 不是闰年
}
}
int days_in_month(int month, int year) {
switch(month) {
case 1: // January
case 3: // March
case 5: // May
case 7: // July
case 8: // August
case 10: // October
case 12: // December
return 31;
case 4: // April
case 6: // June
case 9: // September
case 11: // November
return 30;
case 2: // February
if (is_leap_year(year)) {
return 29;
} else {
return 28;
}
default: // 非法月份
printf("Invalid month entered.\n");
return -1; // 或者你可以选择抛出错误或返回其他值
}
}
int main() {
int year, month;
printf("Enter a year and month (in numbers): ");
scanf("%d %d", &year, &month);
if (month >= 1 && month <= 12) { // 检查月份是否合法
int days = days_in_month(month, year);
if (days != -1) {
printf("There are %d days in %d-%02d.\n", days, year, month);
} else {
// 如果这里打印,说明已经处理了非法月份的情况
// 打印错误信息
}
} else {
printf("Invalid month entered.\n");
}
return 0;
}
```
这个程序首先定义了一个`is_leap_year`函数来检查是否为闰年,然后`days_in_month`函数根据月份返回相应的天数,包括闰年的特殊规则。`main`函数负责获取用户的输入并调用这两个函数。
阅读全文
相关推荐
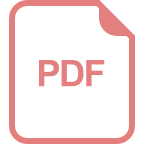
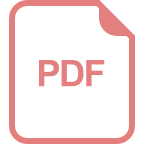














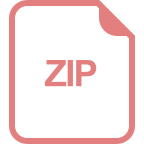
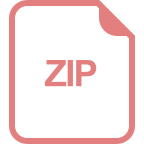