Like functions, generators can also be higher-order. For this problem, we will be writing remainders_generator, which yields a series of generator objects. remainders_generator takes in an integer m, and yields m different generators. The first generator is a generator of multiples of m, i.e. numbers where the remainder is 0. The second is a generator of natural numbers with remainder 1 when divided by m. The last generator yields natural numbers with remainder m - 1 when divided by m. Note that different generators should not influence each other.
时间: 2024-02-24 13:00:33 浏览: 93
Here's a Python implementation of the `remainders_generator` function:
```
def remainders_generator(m):
"""
Generate m different generators that yield numbers with remainders 0 to m-1 when divided by m.
"""
for i in range(m):
yield (x for x in range(i, float('inf'), m))
```
Explanation:
- The `remainders_generator` function takes one argument `m`, the number of generators to yield.
- It uses a `for` loop to generate `m` different generators, one for each remainder from 0 to `m-1`.
- For each remainder `i`, it yields a generator expression `(x for x in range(i, float('inf'), m))`, which generates all natural numbers with remainder `i` when divided by `m`.
- Note that each generator expression is wrapped in parentheses to create a generator object, and that the `float('inf')` argument to `range` creates an unbounded range that generates numbers infinitely.
Here's an example usage of the `remainders_generator` function:
```
>>> gen0, gen1, gen2 = remainders_generator(3)
>>> next(gen0)
0
>>> next(gen1)
1
>>> next(gen2)
2
>>> next(gen0)
3
>>> next(gen1)
4
>>> next(gen2)
5
>>> next(gen0)
6
>>> next(gen1)
7
>>> next(gen2)
8
```
Note that each generator can be used independently, and calling `next` on one generator does not affect the others.
阅读全文
相关推荐
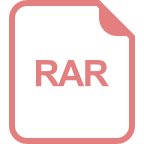
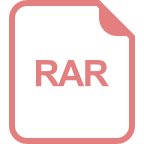

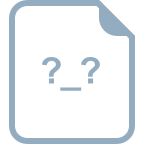




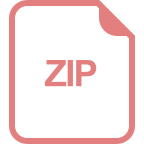
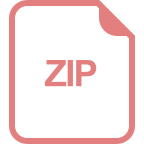
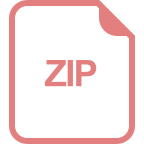
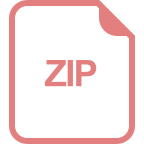