用C#写一段代码,将上位机测试的UDS报文结果和测试时间、是否通过,写入到一个excel中并导出
时间: 2023-12-10 09:42:22 浏览: 38
以下是一个基本的示例,使用OpenXml SDK实现将数据写入Excel文件:
```csharp
using DocumentFormat.OpenXml;
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Spreadsheet;
using System;
using System.Collections.Generic;
using System.IO;
namespace UDSReportToExcel
{
class Program
{
static void Main(string[] args)
{
// 假设这是上位机测试的UDS报文结果
List<TestResult> testResults = new List<TestResult>()
{
new TestResult("01 01", DateTime.Now, true),
new TestResult("02 01", DateTime.Now.AddMinutes(-1), false),
new TestResult("03 01", DateTime.Now.AddMinutes(-2), true),
new TestResult("04 01", DateTime.Now.AddMinutes(-3), false),
};
// 创建一个Excel文件并写入数据
string fileName = "UDS_Test_Results.xlsx";
using (SpreadsheetDocument document = SpreadsheetDocument.Create(fileName, SpreadsheetDocumentType.Workbook))
{
WorkbookPart workbookPart = document.AddWorkbookPart();
workbookPart.Workbook = new Workbook();
WorksheetPart worksheetPart = workbookPart.AddNewPart<WorksheetPart>();
worksheetPart.Worksheet = new Worksheet(new SheetData());
Sheets sheets = workbookPart.Workbook.AppendChild(new Sheets());
Sheet sheet = new Sheet() { Id = workbookPart.GetIdOfPart(worksheetPart), SheetId = 1, Name = "Test Results" };
sheets.Append(sheet);
SheetData sheetData = worksheetPart.Worksheet.GetFirstChild<SheetData>();
// 写入表头
Row headerRow = new Row();
headerRow.Append(
new Cell() { CellValue = new CellValue("Test Result"), DataType = CellValues.String },
new Cell() { CellValue = new CellValue("Test Time"), DataType = CellValues.String },
new Cell() { CellValue = new CellValue("Pass/Fail"), DataType = CellValues.String }
);
sheetData.AppendChild(headerRow);
// 写入数据
foreach (TestResult testResult in testResults)
{
Row dataRow = new Row();
dataRow.Append(
new Cell() { CellValue = new CellValue(testResult.Result), DataType = CellValues.String },
new Cell() { CellValue = new CellValue(testResult.Time.ToString("yyyy-MM-dd HH:mm:ss")), DataType = CellValues.String },
new Cell() { CellValue = new CellValue(testResult.Passed ? "Pass" : "Fail"), DataType = CellValues.String }
);
sheetData.AppendChild(dataRow);
}
workbookPart.Workbook.Save();
}
Console.WriteLine($"数据已写入Excel文件 {fileName} 中。");
}
}
class TestResult
{
public string Result { get; set; }
public DateTime Time { get; set; }
public bool Passed { get; set; }
public TestResult(string result, DateTime time, bool passed)
{
Result = result;
Time = time;
Passed = passed;
}
}
}
```
该示例将测试结果、测试时间和是否通过三个字段写入Excel文件中,并导出到当前目录下的UDS_Test_Results.xlsx文件中。你可以根据自己的需求修改代码。
相关推荐
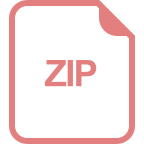
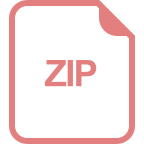














