求解带约束条件的遗传算法Python代码
时间: 2023-11-21 13:43:20 浏览: 105
以下是一个简单的带约束条件的遗传算法的Python代码实现,其中包含一个简单的约束条件函数:
```python
import random
POPULATION_SIZE = 100
GENERATIONS = 50
CROSSOVER_PROBABILITY = 0.8
MUTATION_PROBABILITY = 0.2
ELITISM = True
ELITE_SIZE = 5
LOWER_BOUND = 0
UPPER_BOUND = 10
def fitness(chromosome):
# 计算适应度值
return sum(chromosome)
def constraint(chromosome):
# 约束条件函数,返回 True 表示满足所有约束条件,返回 False 表示不满足
return sum(chromosome) <= 15
def initialize_population():
# 初始化种群
return [[random.uniform(LOWER_BOUND, UPPER_BOUND) for _ in range(10)] for _ in range(POPULATION_SIZE)]
def selection(population):
# 轮盘赌选择
fitness_sum = sum(fitness(chromosome) for chromosome in population)
selection_probabilities = [fitness(chromosome) / fitness_sum for chromosome in population]
return random.choices(population, weights=selection_probabilities, k=2)
def crossover(parents):
# 一点交叉
if random.random() < CROSSOVER_PROBABILITY:
crossover_point = random.randint(0, len(parents[0])-1)
child1 = parents[0][:crossover_point] + parents[1][crossover_point:]
child2 = parents[1][:crossover_point] + parents[0][crossover_point:]
return child1, child2
else:
return parents
def mutation(chromosome):
# 突变,随机将某个基因替换为随机值
if random.random() < MUTATION_PROBABILITY:
mutation_point = random.randint(0, len(chromosome)-1)
chromosome[mutation_point] = random.uniform(LOWER_BOUND, UPPER_BOUND)
return chromosome
def evolve(population):
# 进化
new_population = []
if ELITISM:
# 保留精英
elite = sorted(population, key=fitness, reverse=True)[:ELITE_SIZE]
new_population.extend(elite)
while len(new_population) < POPULATION_SIZE:
parents = selection(population)
children = crossover(parents)
mutated_children = [mutation(child) for child in children]
if constraint(mutated_children[0]):
new_population.append(mutated_children[0])
if constraint(mutated_children[1]):
new_population.append(mutated_children[1])
return new_population
if __name__ == '__main__':
population = initialize_population()
for generation in range(GENERATIONS):
population = evolve(population)
best_chromosome = max(population, key=fitness)
print(f'Generation: {generation+1}, Best fitness: {fitness(best_chromosome)}, Best chromosome: {best_chromosome}')
```
在这个例子中,我们定义了一个目标函数 fitness(chromosome),它计算染色体的适应度值。约束函数 constraint(chromosome) 返回 True 表示染色体满足所有约束条件,返回 False 表示不满足。initialize_population() 函数初始化种群,selection() 函数使用轮盘赌选择进行选择,crossover() 函数进行一点交叉,mutation() 函数进行基因突变。evolve() 函数将这些操作组合在一起来生成新的种群。在每一代中,我们都记录最佳染色体的适应度值和染色体本身。
阅读全文
相关推荐
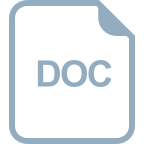
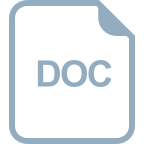
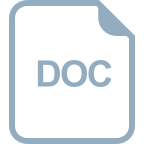
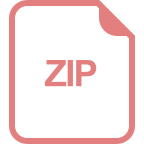
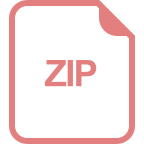
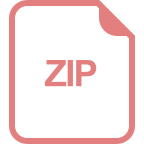
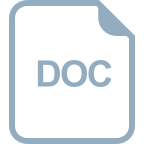











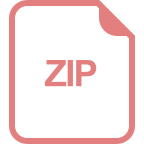