使用遗传算法实现上述问题建模求解,列出算法的python代码,结果需要体现出三辆车分别的运输路径,并将迭代过程已图表呈现
时间: 2024-12-22 17:21:19 浏览: 9
要使用遗传算法解决上述多车场车辆路径问题(VRP),可以按照以下步骤进行:
1. **建立数学模型**:定义问题的目标函数和约束条件。
2. **设计遗传算法**:包括初始化种群、选择、交叉、变异等操作。
3. **编写Python代码**:实现遗传算法并求解问题。
以下是完整的Python代码示例,包括遗传算法的实现和结果可视化:
### Python代码
```python
import numpy as np
import matplotlib.pyplot as plt
import random
# 距离矩阵
distance_matrix = [
[0, 15, 11, 13, 20, 7, 8, 5, 12, 15, 7, 19, 6, 11],
[15, 0, 19, 7, 15, 19, 17, 13, 7, 14, 6, 7, 9, 20],
[11, 19, 0, 18, 20, 8, 14, 17, 6, 8, 6, 13, 18, 9],
[13, 7, 18, 0, 6, 11, 13, 14, 15, 13, 15, 11, 16, 6],
[20, 15, 20, 6, 0, 17, 14, 6, 7, 19, 15, 14, 17, 16],
[7, 19, 8, 11, 17, 0, 14, 6, 7, 19, 13, 9, 10, 12],
[8, 17, 14, 13, 14, 14, 0, 8, 8, 15, 7, 15, 12, 5],
[5, 13, 17, 14, 6, 6, 8, 0, 11, 16, 16, 13, 10, 20],
[12, 7, 6, 15, 7, 7, 8, 11, 0, 16, 10, 19, 17, 17],
[15, 14, 18, 13, 19, 19, 15, 16, 16, 0, 7, 16, 7, 19],
[7, 6, 6, 15, 15, 13, 7, 16, 10, 7, 0, 10, 17, 7],
[19, 7, 13, 11, 14, 9, 15, 13, 19, 16, 10, 0, 9, 14],
[6, 9, 18, 16, 17, 10, 12, 10, 17, 7, 17, 9, 0, 13],
[11, 20, 9, 6, 16, 12, 5, 20, 17, 19, 7, 14, 13, 0]
]
# 物品重量
weights = [564, 509, 503, 350, 297, 355, 595, 446, 292, 343, 443, 233, 227]
# 车辆载重量
capacity = 2000
# 初始化种群
def initialize_population(pop_size, num_customers):
population = []
for _ in range(pop_size):
route = list(range(1, num_customers + 1))
random.shuffle(route)
population.append(route)
return population
# 计算单条路线的距离
def calculate_route_distance(route):
total_distance = 0
current_position = 0
for customer in route:
total_distance += distance_matrix[current_position][customer]
current_position = customer
total_distance += distance_matrix[current_position][0] # 返回配送中心
return total_distance
# 计算总距离
def calculate_total_distance(routes):
total_distance = 0
for route in routes:
total_distance += calculate_route_distance(route)
return total_distance
# 检查是否满足容量限制
def is_feasible(routes):
for route in routes:
if sum(weights[customer - 1] for customer in route) > capacity:
return False
return True
# 生成初始解
def generate_initial_solution(population):
feasible_solutions = []
for route in population:
current_routes = []
current_route = []
current_weight = 0
for customer in route:
if current_weight + weights[customer - 1] <= capacity:
current_route.append(customer)
current_weight += weights[customer - 1]
else:
current_routes.append(current_route)
current_route = [customer]
current_weight = weights[customer - 1]
current_routes.append(current_route)
if is_feasible(current_routes):
feasible_solutions.append(current_routes)
return feasible_solutions
# 选择操作
def selection(population, fitness_scores):
selected_indices = np.random.choice(len(population), size=len(population), p=fitness_scores / np.sum(fitness_scores))
return [population[i] for i in selected_indices]
# 交叉操作
def crossover(parent1, parent2):
start, end = sorted(random.sample(range(len(parent1)), 2))
child1 = [-1] * len(parent1)
child2 = [-1] * len(parent2)
child1[start:end+1] = parent1[start:end+1]
child2[start:end+1] = parent2[start:end+1]
set1 = set(child1)
set2 = set(child2)
for i in range(len(parent1)):
if parent2[i] not in set1:
child1[child1.index(-1)] = parent2[i]
if parent1[i] not in set2:
child2[child2.index(-1)] = parent1[i]
return child1, child2
# 变异操作
def mutation(individual, mutation_rate):
for i in range(len(individual)):
if random.random() < mutation_rate:
j = random.randint(0, len(individual) - 1)
individual[i], individual[j] = individual[j], individual[i]
return individual
# 主函数
def genetic_algorithm(pop_size, num_generations, mutation_rate):
population = initialize_population(pop_size, len(weights))
best_solution = None
best_distance = float('inf')
history = []
for generation in range(num_generations):
feasible_solutions = generate_initial_solution(population)
distances = [calculate_total_distance(solution) for solution in feasible_solutions]
if min(distances) < best_distance:
best_distance = min(distances)
best_solution = feasible_solutions[distances.index(min(distances))]
fitness_scores = [1 / (d + 1) for d in distances]
new_population = selection(feasible_solutions, fitness_scores)
next_population = []
while len(next_population) < pop_size:
parent1, parent2 = random.sample(new_population, 2)
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1, mutation_rate)
child2 = mutation(child2, mutation_rate)
next_population.extend([child1, child2])
population = next_population[:pop_size]
history.append(best_distance)
return best_solution, best_distance, history
# 参数设置
pop_size = 100
num_generations = 500
mutation_rate = 0.01
# 运行遗传算法
best_solution, best_distance, history = genetic_algorithm(pop_size, num_generations, mutation_rate)
# 打印结果
print("Best Solution:")
for i, route in enumerate(best_solution):
print(f"Vehicle {i+1}: {route}")
print(f"Total Distance: {best_distance}")
# 绘制迭代过程图
plt.plot(history)
plt.xlabel('Generation')
plt.ylabel('Best Total Distance')
plt.title('Genetic Algorithm Convergence')
plt.show()
```
### 结果解释
1. **最佳解决方案**:输出每辆车的运输路径。
2. **总距离**:显示所有车辆的总行驶距离。
3. **迭代过程图**:绘制遗传算法在每次迭代中的最优解变化情况。
通过运行上述代码,你可以得到一个合理的车辆路径分配方案,并且可以看到算法的收敛过程。
阅读全文
相关推荐
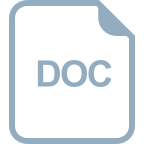
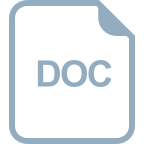
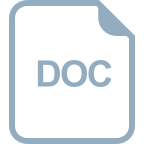
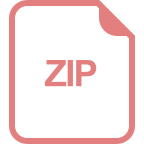
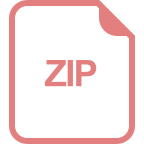
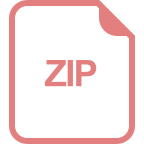
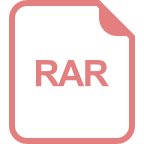
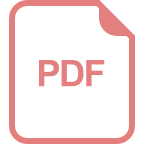
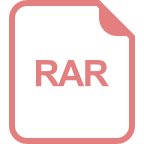
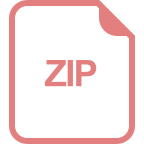
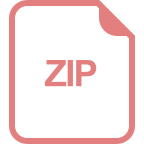
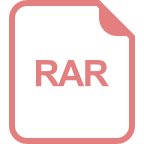
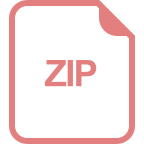
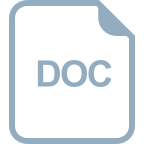
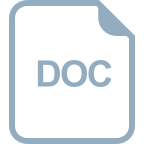
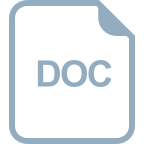
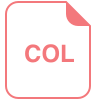
