提供一个带有约束条件的目标优化算法的遗传算法求解代码框架
时间: 2024-03-19 18:45:22 浏览: 23
以下是一个基于遗传算法求解带有约束条件的目标优化问题的代码框架:
```python
import numpy as np
import random
# 定义目标函数,返回函数值和约束是否满足的布尔值
def objective_function(x):
# TODO: 实现目标函数
return f, is_feasible
# 定义遗传算法参数
population_size = 100
chromosome_length = 10
crossover_rate = 0.8
mutation_rate = 0.1
max_generation = 100
# 初始化种群
population = np.random.randint(0, 2, size=(population_size, chromosome_length))
# 迭代进化
for generation in range(max_generation):
# 计算种群适应度
fitness = np.zeros(population_size)
feasibility = np.zeros(population_size, dtype=bool)
for i, chromosome in enumerate(population):
fitness[i], feasibility[i] = objective_function(chromosome)
# 选择
selection_probability = fitness / np.sum(fitness)
selected_indices = np.random.choice(population_size, size=population_size, p=selection_probability)
selected_population = population[selected_indices]
# 交叉
for i in range(0, population_size, 2):
if random.random() < crossover_rate:
crossover_point = random.randint(1, chromosome_length - 1)
selected_population[i, crossover_point:], selected_population[i+1, crossover_point:] = \
selected_population[i+1, crossover_point:], selected_population[i, crossover_point:]
# 变异
for i in range(population_size):
if random.random() < mutation_rate:
mutation_point = random.randint(0, chromosome_length - 1)
selected_population[i, mutation_point] = 1 - selected_population[i, mutation_point]
# 更新种群
population = selected_population
# 输出当前进化代数和最优解
best_index = np.argmax(fitness)
best_chromosome = population[best_index]
best_fitness = fitness[best_index]
print("Generation {}: Best fitness = {}".format(generation, best_fitness))
```
在这个代码框架中,我们使用了二进制编码表示染色体,并通过 `objective_function` 函数计算染色体对应的函数值和约束是否满足。在每一代中,我们首先计算种群适应度,然后进行选择、交叉和变异操作,并更新种群。最后,输出当前进化代数和最优解。
需要注意的是,这个代码框架只提供了一个基本的遗传算法实现,实际应用中可能需要根据具体的问题进行调整和优化。例如,可以使用更复杂的遗传算子、改进选择策略、引入多目标优化等方法来提高算法性能。
相关推荐
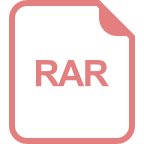
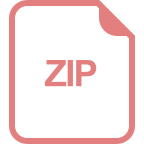
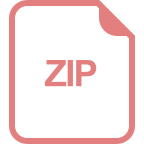













