string text;text = std::to_string(lineInfo.value);如果lineInfo.value是2.0,那么执行完以后我希望text也是2.0,而不是2.000000,有那些方法可以实现
时间: 2024-09-28 11:03:37 浏览: 10
在C++中,如果你希望将浮点数转换成字符串时不包含过多的小数位,可以使用`std::fixed`和`std::setprecision`来控制输出精度。`std::fixed`会使得小数部分始终使用固定数量的数字表示,并且`std::setprecision`用于设置最多显示多少位小数。例如:
```cpp
#include <iostream>
#include <sstream>
std::string toStringWithFixedPrecision(double value) {
std::ostringstream oss;
oss << std::fixed << std::setprecision(2); // 设置两位小数
oss << value;
return oss.str();
}
int main() {
double lineInfoValue = 2.0;
std::string text = toStringWithFixedPrecision(lineInfoValue);
std::cout << "Text after conversion: " << text << std::endl; // 输出 "2.00"
return 0;
}
```
在这个例子中,即使`lineInfo.value`是2.000000,转换后的文本也会是"2.00"。
相关问题
struct SMS { int index; std::string state; std::string sender; std::string timestamp; std::string message; }; std::vector<SMS> smsList; bool serial_port::serial_port_write_read_at_bySMS(const std::string &at_command, const std::string &resp_prefix, std::vector<SMS>& smsList) { std::vector<std::string> read_lines; bool ret_value = false; pthread_mutex_lock(&read_write_mutex); if (!serial_port_write_at_cmd(at_command.c_str())) { goto END; } while (true) { int index = -1, n = -1; char state[32] = {}, phone_num[32] = {}, phone_time[64] = {}; read_lines = serial_port_read_multiple_lines(); for (size_t i = 0; i < read_lines.size(); i++) { //LOG_F(INFO, "read one line from serial: %s", read_line); if (read_lines[i].find("AT") != std::string::npos || read_lines[i].find("at") != std::string::npos) { //Do nothing } else if (read_lines[i].find("OK") != std::string::npos) { //LOG_F(INFO, "find final response OK"); ret_value = true; break; } else if (read_lines[i].find("ERROR") != std::string::npos || read_lines[i].find("+CME ERROR") != std::string::npos) { //LOG_F(INFO, "find final response ERROR"); ret_value = false; break; } else if (read_lines[i].find("+CMGL") != std::string::npos) { LOG_F(INFO, "response_data[%d]: %s", i, read_lines[i]); sscanf(read_lines[i], R"(+CMGL: %d,"%s","%s","%s")", &n, state, phone_num, phone_time); LOG_F(INFO, "n: %d, state: %s, phone_num: %s, phone_time: %s", n, state, phone_num, phone_time); SMS sms; index = i; sms.index = index; sms.state = state; sms.sender = phone_num; sms.timestamp = phone_time; if (read_lines[i].find('\n') != std::string::npos) { sms.message = read_lines[i+1]; } smsList.push_back(sms); // break; } else { LOG_F(INFO, "response_data[%d]->message: %s", i, read_lines[i]); // 继续往下读一行 } } if (index == -1) { break; // 未找到新的响应,退出循环 } } END: pthread_mutex_unlock(&read_write_mutex); return ret_value; } 这段代码有问题吗?如何改正
这段代码存在一些问题。以下是一些可能需要改正的问题:
1. 在循环中,`index`变量应该在每次循环开始时被设置为-1,以便判断是否找到了新的响应。目前,`index`只在找到"+CMGL"响应时被设置为有效值,但是没有在未找到新响应时重置为-1。
2. 在读取响应行时,使用`find()`函数来检查字符串是否包含特定内容。这种方法可能导致误判,因为只要字符串中包含目标内容即可,而不需要完全匹配。建议使用`==`运算符进行精确匹配。
3. 在读取短信消息内容时,使用了错误的索引。根据代码中的逻辑,应该是读取`read_lines[i+1]`作为短信消息内容,而不是`read_lines[i]`。
4. 在读取短信消息内容之前,应该先检查索引是否超出了`read_lines`的范围,避免访问越界。
5. 在读取短信消息内容之后,应该将索引设置为下一个未读取的响应行的索引,以便下一次循环时能够正确读取下一个短信。
下面是修改后的代码示例:
```cpp
bool serial_port::serial_port_write_read_at_bySMS(const std::string &at_command,
const std::string &resp_prefix,
std::vector<SMS>& smsList)
{
std::vector<std::string> read_lines;
bool ret_value = false;
pthread_mutex_lock(&read_write_mutex);
if (!serial_port_write_at_cmd(at_command.c_str()))
{
goto END;
}
int index = -1; // 初始化索引为-1
while (true)
{
int n = -1;
char state[32] = {}, phone_num[32] = {}, phone_time[64] = {};
read_lines = serial_port_read_multiple_lines();
for (size_t i = 0; i < read_lines.size(); i++)
{
// LOG_F(INFO, "read one line from serial: %s", read_lines[i].c_str());
if (read_lines[i] == "AT" || read_lines[i] == "at")
{
// Do nothing
}
else if (read_lines[i] == "OK")
{
// LOG_F(INFO, "find final response OK");
ret_value = true;
break;
}
else if (read_lines[i] == "ERROR" || read_lines[i] == "+CME ERROR")
{
// LOG_F(INFO, "find final response ERROR");
ret_value = false;
break;
}
else if (read_lines[i].find("+CMGL") != std::string::npos)
{
LOG_F(INFO, "response_data[%zu]: %s", i, read_lines[i].c_str());
sscanf(read_lines[i].c_str(),
R"(+CMGL: %d,"%s","%s","%s")", &n, state, phone_num, phone_time);
LOG_F(INFO, "n: %d, state: %s, phone_num: %s, phone_time: %s", n, state, phone_num, phone_time);
SMS sms;
index = i;
sms.index = index;
sms.state = state;
sms.sender = phone_num;
sms.timestamp = phone_time;
if (i + 1 < read_lines.size() && read_lines[i + 1].find('\n') != std::string::npos)
{
sms.message = read_lines[i + 1];
index = i + 1; // 设置索引为下一个未读取的响应行的索引
}
smsList.push_back(sms);
}
else
{
LOG_F(INFO, "response_data[%zu]->message: %s", i, read_lines[i].c_str());
// 继续往下读一行
}
}
if (index == -1)
{
break; // 未找到新的响应,退出循环
}
}
END:
pthread_mutex_unlock(&read_write_mutex);
return ret_value;
}
```
请注意,此处仅提供了一种修改方案,具体修改还需要根据实际需求和代码逻辑进行调整。
struct SMS { int index; std::string state; std::string sender; std::string timestamp; std::string message; }; std::vector<SMS> smsList; bool serial_port::serial_port_write_read_at_bySMS(const std::string &at_command, const std::string &resp_prefix, std::vector<SMS>& smsList) { std::vector<std::string> read_lines; bool ret_value = false; pthread_mutex_lock(&read_write_mutex); if (!serial_port_write_at_cmd(at_command.c_str())) { goto END; } int index = -1 while (true) { int n = -1; char state[32] = {}, phone_num[32] = {}, phone_time[64] = {}; read_lines = serial_port_read_multiple_lines(); for (size_t i = 0; i < read_lines.size(); i++) { //LOG_F(INFO, "read one line from serial: %s", read_lines[i].c_str()); if (read_lines[i] == "AT" || read_lines[i] == "at") { //Do nothing } else if (read_lines[i] == "OK") { //LOG_F(INFO, "find final response OK"); ret_value = true; break; } else if (read_lines[i] == "ERROR" || read_lines[i] == "+CME ERROR") { //LOG_F(INFO, "find final response ERROR"); ret_value = false; break; } else if (read_lines[i].find("+CMGL") != std::string::npos) { LOG_F(INFO, "response_data[%zu]: %s", i, read_lines[i].c_str()); sscanf(read_lines[i].c_str(), R"(+CMGL: %d,"%s","%s","%s")", &n, state, phone_num, phone_time); LOG_F(INFO, "n: %d, state: %s, phone_num: %s, phone_time: %s", n, state, phone_num, phone_time); SMS sms; index = i; sms.index = index; sms.state = state; sms.sender = phone_num; sms.timestamp = phone_time; if (i + 1 < read_lines.size() && read_lines[i + 1].find('\n') != std::string::npos) { sms.message = read_lines[i+1]; index = i + 1; // 设置索引为下一个未读取的响应行的索引 } smsList.push_back(sms); } else { LOG_F(INFO, "response_data[%zu]->message: %s", i, read_lines[i].c_str()); // 继续往下读一行 } } if (index == -1) { break; // 未找到新的响应,退出循环 } } END: pthread_mutex_unlock(&read_write_mutex); return ret_value; } 如果要调用这个api应该怎么写
要调用`serial_port_write_read_at_bySMS`函数并使用它的返回值和`smsList`,可以按照以下方式进行编写:
```cpp
std::vector<SMS> smsList; // 声明并初始化 SMS 列表
// 调用 serial_port_write_read_at_bySMS 函数
bool result = serial_port_write_read_at_bySMS(at_command, resp_prefix, smsList);
// 检查结果并处理 smsList
if (result) {
// 成功执行,处理 smsList
for (const auto& sms : smsList) {
// 在这里使用 sms 对象的属性进行处理
// 例如,sms.index, sms.state, sms.sender, sms.timestamp, sms.message
}
}
else {
// 执行失败,根据需要进行错误处理
}
```
在上述代码中,`at_command` 和 `resp_prefix` 是作为参数传递给 `serial_port_write_read_at_bySMS` 函数的输入值。调用函数后,根据返回的 `result` 值来判断操作是否成功。如果成功,可以遍历 `smsList` 来处理每个 SMS 对象的属性。如果失败,则可以根据需要进行错误处理。
请注意,在调用该函数之前,需要确保 `at_command` 和 `resp_prefix` 的值已经被正确设置,并且 `smsList` 是一个有效的、空的 `std::vector<SMS>` 对象。
阅读全文
相关推荐
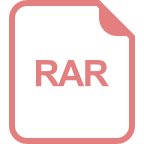
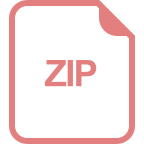


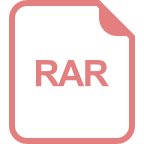
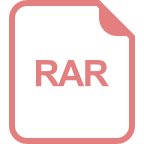
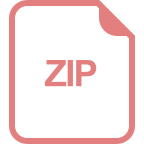
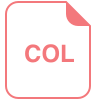
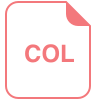
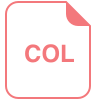
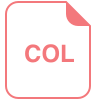
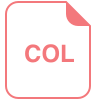
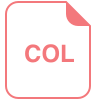
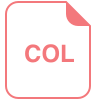
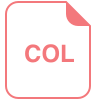
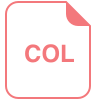
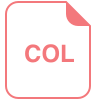