用C语言编写如下程序:编写二个猜数的小游戏,在程序里设定一个数,运行程序让别人猜。如果猜的教比设定的数大,给出“大了”的提示,如果猜的数比设定的数小,则给出“小了”的提示,设定有5次机会,5次内清中给出“清中”的那示,否则提示“你输了”
时间: 2024-05-05 14:15:57 浏览: 219
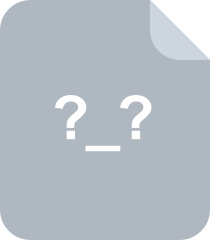
猜数游戏,c语言制作的小游戏。
```c
#include <stdio.h>
int main() {
int answer = 42; // 设定的数
int guess; // 猜的数
int chance = 5; // 剩余机会
printf("猜数小游戏开始了!\n");
printf("你有5次机会猜出我设定的数,范围是1到100。\n");
while (chance > 0) {
printf("请输入你猜的数:");
scanf("%d", &guess);
if (guess == answer) {
printf("恭喜你,猜对了!\n");
break;
} else if (guess > answer) {
printf("大了,再猜一次!\n");
} else {
printf("小了,再猜一次!\n");
}
chance--;
if (chance == 0) {
printf("你输了,正确答案是%d。\n", answer);
break;
} else {
printf("你还有%d次机会。\n", chance);
}
}
return 0;
}
```
阅读全文
相关推荐
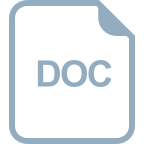








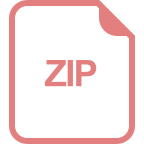





