用C语言定义一个虚数,实部和虚部分开储存和计算的函数并可执行
时间: 2024-03-01 20:54:10 浏览: 21
好的,以下是用C语言定义一个虚数,实部和虚部分开储存和计算的函数的代码:
```c
#include <stdio.h>
// 定义虚数结构体
typedef struct {
double real; // 实部
double imag; // 虚部
} complex;
// 定义计算虚数模长的函数
double abs(complex c) {
return sqrt(c.real * c.real + c.imag * c.imag);
}
// 定义计算虚数共轭的函数
complex conjugate(complex c) {
complex res;
res.real = c.real;
res.imag = -c.imag;
return res;
}
// 定义计算虚数加法的函数
complex add(complex c1, complex c2) {
complex res;
res.real = c1.real + c2.real;
res.imag = c1.imag + c2.imag;
return res;
}
// 定义计算虚数减法的函数
complex subtract(complex c1, complex c2) {
complex res;
res.real = c1.real - c2.real;
res.imag = c1.imag - c2.imag;
return res;
}
// 定义计算虚数乘法的函数
complex multiply(complex c1, complex c2) {
complex res;
res.real = c1.real * c2.real - c1.imag * c2.imag;
res.imag = c1.real * c2.imag + c1.imag * c2.real;
return res;
}
// 定义计算虚数除法的函数
complex divide(complex c1, complex c2) {
complex res;
double denominator = c2.real * c2.real + c2.imag * c2.imag;
res.real = (c1.real * c2.real + c1.imag * c2.imag) / denominator;
res.imag = (c1.imag * c2.real - c1.real * c2.imag) / denominator;
return res;
}
int main() {
// 定义并初始化两个虚数
complex c1 = {1.0, 2.0};
complex c2 = {3.0, 4.0};
// 计算并输出两个虚数的模长
printf("The absolute value of c1 is %lf\n", abs(c1));
printf("The absolute value of c2 is %lf\n", abs(c2));
// 计算并输出两个虚数的共轭
complex conj_c1 = conjugate(c1);
complex conj_c2 = conjugate(c2);
printf("The conjugate of c1 is %lf + %lfi\n", conj_c1.real, conj_c1.imag);
printf("The conjugate of c2 is %lf + %lfi\n", conj_c2.real, conj_c2.imag);
// 计算并输出两个虚数的加法
complex sum = add(c1, c2);
printf("The sum of c1 and c2 is %lf + %lfi\n", sum.real, sum.imag);
// 计算并输出两个虚数的减法
complex diff = subtract(c1, c2);
printf("The difference between c1 and c2 is %lf + %lfi\n", diff.real, diff.imag);
// 计算并输出两个虚数的乘法
complex prod = multiply(c1, c2);
printf("The product of c1 and c2 is %lf + %lfi\n", prod.real, prod.imag);
// 计算并输出两个虚数的除法
complex quot = divide(c1, c2);
printf("The quotient of c1 and c2 is %lf + %lfi\n", quot.real, quot.imag);
return 0;
}
```
以上代码定义了一个虚数结构体,以及计算虚数模长、虚数共轭、虚数加减乘除的函数,并在主函数中演示了这些函数的用法。可以通过编译并运行这段代码来进行测试。
相关推荐
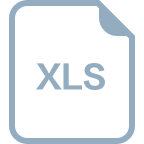














