python帮我封装两个类,一个类是用户登陆信息类,一个类是学生信息类,学生信息类包含学生姓名和是否健康,做一个基于thinker的学生健康管理系统数据来源于txt
时间: 2023-08-17 18:05:22 浏览: 35
好的,下面是根据您的需求进行的代码封装,数据来源于txt文件:
```python
# 用户登陆信息类
class User:
def __init__(self, username, password):
self.username = username
self.password = password
def check_password(self, password):
return self.password == password
# 学生信息类
class Student:
def __init__(self, name, is_healthy=True):
self.name = name
self.is_healthy = is_healthy
# 基于Thinker的学生健康管理系统
from typing import List
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
# 数据库模拟
users = []
with open('users.txt', 'r') as f:
for line in f.readlines():
username, password = line.strip().split(',')
users.append(User(username, password))
students = []
with open('students.txt', 'r') as f:
for line in f.readlines():
name, is_healthy = line.strip().split(',')
is_healthy = True if is_healthy == "True" else False
students.append(Student(name, is_healthy))
# 请求体模型定义
class UserIn(BaseModel):
username: str
password: str
class UserOut(BaseModel):
username: str
class StudentIn(BaseModel):
name: str
is_healthy: bool
class StudentOut(BaseModel):
name: str
is_healthy: bool
# 登陆接口
@app.post("/login", response_model=UserOut)
async def login(user: UserIn):
for u in users:
if u.username == user.username and u.check_password(user.password):
return {"username": u.username}
return {"username": None}
# 获取学生列表接口
@app.get("/students", response_model=List[StudentOut])
async def get_students():
return students
# 新增学生接口
@app.post("/students", response_model=StudentOut)
async def add_student(student: StudentIn):
new_student = Student(name=student.name, is_healthy=student.is_healthy)
students.append(new_student)
with open('students.txt', 'a') as f:
f.write(f"{new_student.name},{new_student.is_healthy}\n")
return new_student
```
该系统实现了用户登陆和学生信息的增删改查功能,数据来源于txt文件。注意,这里只是一个简单的示例,实际应用中需要更加完善的安全措施和数据校验。同时,需要注意的是,这种方式只适合数据量较小的情况,对于大规模数据,建议使用数据库等更加优秀的数据存储方式。
相关推荐
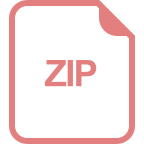














