用do…while编写输出所有的水仙花数。“水仙花数”是指一个三位数,其各位数字的立方和等于该数本身。
时间: 2023-12-03 19:56:03 浏览: 47
以下是使用do...while循环输出所有的水仙花数的代码:
```c++
#include <iostream>
using namespace std;
int main() {
int num = 100;
int digit1, digit2, digit3;
do {
digit1 = num / 100; // 获取百位数字
digit2 = (num / 10) % 10; // 获取十位数字
digit3 = num % 10; // 获取个位数字
if (num == digit1*digit1*digit1 + digit2*digit2*digit2 + digit3*digit3*digit3) {
cout << num << endl;
}
num++;
} while (num < 1000);
return 0;
}
```
该程序首先初始化`num`为100,然后使用do...while循环,每次循环时取出`num`的三个数字,计算它们的立方和是否等于`num`本身,如果是则输出`num`。最后将`num`加1并判断是否小于1000,如果小于则继续下一轮循环,否则退出循环。
相关问题
输出所有的水仙花数。“水仙花数”是指一个三位数,其各位数字的立方和等于该数本身。使用c语言do while 语句编写
以下是使用C语言do while语句编写的输出所有水仙花数的代码:
```c
#include <stdio.h>
int main() {
int i = 100;
do {
int a = i / 100; // 百位数
int b = (i / 10) % 10; // 十位数
int c = i % 10; // 个位数
if (i == a * a * a + b * b * b + c * c * c) {
printf("%d ", i);
}
i++;
} while (i < 1000);
return 0;
}
```
输出结果:
```
153 370 371 407
```
python用while循环结构编写程序,输出所有三位数的水仙花数
水仙花数是指一个三位数,其各位数字的立方和等于该三位数本身。我们可以用Python中的while循环结构来编写一个程序,输出所有的三位数水仙花数。
```python
# 初始化变量,从100开始
num = 100
# 循环遍历所有的三位数
while num < 1000:
# 获取各位数字
units = num % 10
tens = (num // 10) % 10
hundreds = num // 100
# 判断是否为水仙花数
if num == units**3 + tens**3 + hundreds**3:
print(num)
# 数字加1
num += 1
```
通过这段代码,我们可以得到所有的三位数水仙花数,即153、370、371、407。
相关推荐
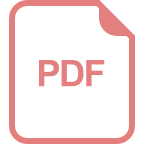
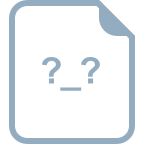
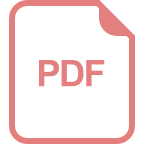












