{ //pcm数据处理 val file = File(inPcmFile) try { val inputStream = FileInputStream(file) val bs = BufferedInputStream(inputStream) var bytesBuffer = ByteArray(block) var outByteBuffer = ByteArray(block * 10); while (true) { val readSize = bs.read(bytesBuffer) val outSampleCount = IntArray(1) val sampleCount = block / bytePerSample Log.e("kuwolog","bytesBuffer:"+bytesBuffer.size+ "sampleCount:"+sampleCount ) SuperSoundJni.supersound_hrs_process_in(mHiresInst, bytesBuffer, sampleCount, outSampleCount) if (outSampleCount[0] > 0) { val outBytes = outSampleCount[0] * bytePerSample if (outByteBuffer.size < outBytes) { outByteBuffer = ByteArray(outBytes); } Log.e("kuwolog","outByteBuffer:"+outByteBuffer.size+ " outSampleCount[0]:"+ outSampleCount[0] +"\n"+outPcmPath); SuperSoundJni.supersound_hrs_process_out(mHiresInst, outByteBuffer, outSampleCount[0], outSampleCount) outByteBuffer?.let { save2File(it, outBytes, outPcmPath) } } if (readSize < block || readSize == 0) break } } catch (e: IOException) { } SuperSoundJni.supersound_hrs_destroy_inst(mHiresInst) }
时间: 2024-02-10 11:32:40 浏览: 14
这段代码是用 Kotlin 编写的,主要是对 PCM 数据进行处理,其中调用了 SuperSoundJni 库中的 supersound_hrs_process_in 和 supersound_hrs_process_out 方法。在处理 PCM 数据的过程中,读取了一个输入 PCM 文件,将其分块读取,然后将读取到的数据传递给 SuperSoundJni 库进行处理,处理结果写入一个输出 PCM 文件中。在处理完成后,调用了 supersound_hrs_destroy_inst 方法来销毁 SuperSoundJni 实例。
相关问题
kotlin 将 File 文件转换为 MultipartFile 文件
在 Kotlin 中,可以使用 Spring 的 MultipartFile 类来表示上传的文件,并且这个类可以从文件系统中的 File 对象中创建。下面是一个示例代码:
```kotlin
import org.springframework.web.multipart.MultipartFile
import java.io.File
import java.io.FileInputStream
import java.io.IOException
fun fileToMultipart(file: File): MultipartFile {
val inputStream = FileInputStream(file)
return object : MultipartFile {
override fun getName(): String = file.name
override fun getOriginalFilename(): String = file.name
override fun getContentType(): String = "application/octet-stream"
override fun isEmpty(): Boolean = file.length() == 0L
override fun getSize(): Long = file.length()
override fun getBytes(): ByteArray = file.readBytes()
override fun getInputStream(): FileInputStream = inputStream
override fun transferTo(dest: File) {
file.copyTo(dest)
}
}
}
```
这个函数接受一个 File 对象作为参数,并返回一个 MultipartFile 对象。这个函数创建了一个匿名的 MultipartFile 对象,它实现了 MultipartFile 接口的所有方法。在这个匿名对象中,我们使用了 File 对象的一些方法来实现 MultipartFile 接口中的方法,比如 getName,getSize 和 getBytes。最后,我们使用 file.copyTo(dest) 方法来将文件复制到目标位置。
使用这个函数可以将 File 对象转换为 MultipartFile 对象,然后可以将它传递给 Spring MVC 的处理器方法或者其他需要 MultipartFile 对象的方法。
在安卓中用kotlin如何使用数据库存图片
在 Android 中使用 Kotlin 存储图片到数据库可以采用以下步骤:
1. 创建一个继承自 RoomDatabase 的抽象类,定义一个用于访问数据库的 DAO 接口。
```kotlin
@Database(entities = [ImageEntity::class], version = 1)
abstract class MyDatabase : RoomDatabase() {
abstract fun imageDao(): ImageDao
}
```
2. 创建一个实体类 ImageEntity,用于表示图片的信息,包括图片的 ID、名称、路径等。
```kotlin
@Entity(tableName = "images")
data class ImageEntity(
@PrimaryKey(autoGenerate = true)
val id: Int,
val name: String,
val path: String
)
```
3. 创建一个 DAO 接口 ImageDao,定义用于访问数据库的方法,包括插入图片、删除图片、查询所有图片等。
```kotlin
@Dao
interface ImageDao {
@Insert(onConflict = OnConflictStrategy.REPLACE)
fun insert(image: ImageEntity)
@Delete
fun delete(image: ImageEntity)
@Query("SELECT * FROM images")
fun getAll(): LiveData<List<ImageEntity>>
}
```
4. 在需要存储图片时,将图片转换成字节数组,并插入到数据库中。
```kotlin
val file = File(imagePath)
val inputStream = FileInputStream(file)
val buffer = ByteArray(file.length().toInt())
inputStream.read(buffer)
val imageBytes = Base64.encodeToString(buffer, Base64.NO_WRAP)
val imageEntity = ImageEntity(0, imageName, imageBytes)
myDatabase.imageDao().insert(imageEntity)
```
5. 在需要显示图片时,从数据库中查询图片信息,并将字节数组转换成 Bitmap。
```kotlin
val imageEntity = myDatabase.imageDao().getById(imageId)
val imageBytes = Base64.decode(imageEntity.path, Base64.NO_WRAP)
val bitmap = BitmapFactory.decodeByteArray(imageBytes, 0, imageBytes.size)
```
注意:存储图片到数据库可能会导致数据库占用过多空间,建议仅存储图片的路径或 URI,图片本身保存在文件系统中。
相关推荐
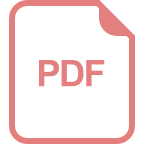
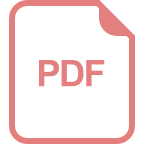
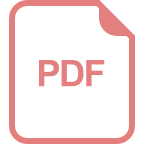









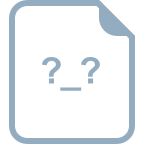
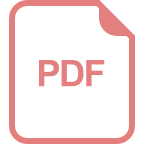
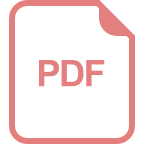