ListView{ id:listView anchors.left: parent.left anchors.right: parent.right anchors.top: row.bottom anchors.bottom: parent.bottom clip: true spacing:0 snapMode: ListView.SnapToItem ScrollBar.vertical: ScrollBar{ id:ver_scrollbar visible:true } model: ListModel{ id:listModel ListElement{ time:"2021-03-12\n12:21:31" floor:1 } } delegate: Rectangle{ id:infoRec implicitHeight: 640 implicitWidth: 480 width: listView.width height: 120 Rectangle{ id:dim anchors.fill: parent color: "grey" opacity: 0.3 z:300 visible: tableItem.hightLightIndex == index } //显示时间与层数的 Rectangle { id: timeRec width: parent.width color: index % 2?tableItem.evenRowColor:tableItem.oddRowColor anchors.left: parent.left anchors.top: parent.top anchors.topMargin: floorRec.height anchors.bottom: parent.bottom Text { id: timeText text: { (time === undefined?errorStr:time)+" "+(floor === undefined?errorStr:floor) } property var adderss : adderss anchors.fill: parent color: "#002949" font.pixelSize: tableItem.timeFontPixelSize font.family: tableItem.contentFontFamily horizontalAlignment: Text.AlignHCenter verticalAlignment: Text.AlignVCenter wrapMode: Text.WrapAnywhere clip: true } } } }添加点击事件
时间: 2024-02-10 14:21:17 浏览: 29
要给 ListView 中的 delegate 添加点击事件,需要在 delegate 元素中添加 MouseArea 元素,并设置其属性来响应鼠标点击事件。例如,可以在 Rectangle 元素中添加一个 MouseArea 元素,设置其 anchors.fill: parent,使其与 Rectangle 元素大小相同,并设置其 onClicked 信号来响应鼠标点击事件,如下所示:
```
delegate: Rectangle {
id: infoRec
implicitHeight: 640
implicitWidth: 480
width: listView.width
height: 120
MouseArea {
anchors.fill: parent
onClicked: {
// 处理鼠标点击事件
console.log("Item clicked:", index, time, floor);
}
}
// 其他元素的定义...
}
```
在 MouseArea 的 onClicked 信号中,可以调用 JavaScript 函数或执行其他操作来处理鼠标点击事件。其中的 index、time、floor 等变量可以通过 ListView 的 model 属性来获取。
相关问题
ListView{ id:listView anchors.left: parent.left anchors.right: parent.right anchors.top: row.bottom anchors.bottom: parent.bottom clip: true spacing:0 snapMode: ListView.SnapToItem ScrollBar.vertical: ScrollBar{ id:ver_scrollbar visible:true } model: ListModel{ id:listModel ListElement{ time:"2021-03-12\n12:21:31" floor:1 } } delegate: Rectangle{ id:infoRec implicitHeight: 640 implicitWidth: 480 width: listView.width height: 120 Rectangle{ id:dim anchors.fill: parent color: "grey" opacity: 0.3 z:300 visible: tableItem.hightLightIndex == index } //时间显示区域 Rectangle { id: timeRec width: 90 color: index % 2?tableItem.evenRowColor:tableItem.oddRowColor anchors.left: parent.left anchors.top: parent.top anchors.topMargin: floorRec.height anchors.bottom: parent.bottom Text { id: timeText text: time === undefined?errorStr:time anchors.fill: parent color: "#002949" font.pixelSize: tableItem.timeFontPixelSize font.family: tableItem.contentFontFamily horizontalAlignment: Text.AlignHCenter verticalAlignment: Text.AlignVCenter wrapMode: Text.WrapAnywhere clip: true function replaceBlank(str){ let newStr = str newStr = newStr.replace(" ","\n") } } } } } 每行打上注释
好的,以下是每行的注释:
```
ListView{ // 定义一个名为 listView 的列表视图控件
id: listView // 控件的唯一标识符
anchors.left: parent.left // 控件与其父控件左对齐
anchors.right: parent.right // 控件与其父控件右对齐
anchors.top: row.bottom // 控件顶部与名为 row 的控件底部对齐
anchors.bottom: parent.bottom // 控件底部与其父控件底部对齐
clip: true // 列表内容超出控件边界时进行裁剪
spacing: 0 // 列表项之间的间距为0
snapMode: ListView.SnapToItem // 当滚动结束时,列表项会自动对齐到最近的项
ScrollBar.vertical: ScrollBar{ // 垂直滚动条
id: ver_scrollbar // 控件的唯一标识符
visible: true // 控件可见
}
model: ListModel{ // 列表数据模型
id: listModel // 控件的唯一标识符
ListElement{ // 列表项元素
time: "2021-03-12\n12:21:31" // 时间属性
floor: 1 // 楼层属性
}
}
delegate: Rectangle{ // 列表项的委托组件
id: infoRec // 控件的唯一标识符
implicitHeight: 640 // 控件的默认高度
implicitWidth: 480 // 控件的默认宽度
width: listView.width // 控件的宽度等于列表视图控件的宽度
height: 120 // 控件的高度为120
Rectangle{ // 控件背景
id: dim // 控件的唯一标识符
anchors.fill: parent // 控件占满父控件
color: "grey" // 控件背景颜色
opacity: 0.3 // 控件背景透明度
z: 300 // 控件的 Z 轴坐标
visible: tableItem.hightLightIndex == index // 根据高亮索引决定控件可见性
}
// 时间显示区域
Rectangle { // 时间显示区域
id: timeRec // 控件的唯一标识符
width: 90 // 控件宽度
color: index % 2 ? tableItem.evenRowColor : tableItem.oddRowColor // 控件背景颜色
anchors.left: parent.left // 控件左对齐
anchors.top: parent.top // 控件顶部对齐
anchors.topMargin: floorRec.height // 控件顶部外边距为楼层显示控件的高度
anchors.bottom: parent.bottom // 控件底部对齐
Text { // 时间文本控件
id: timeText // 控件的唯一标识符
text: time === undefined ? errorStr : time // 控件显示的文本内容
anchors.fill: parent // 控件占满父控件
color: "#002949" // 控件文本颜色
font.pixelSize: tableItem.timeFontPixelSize // 控件文本字体大小
font.family: tableItem.contentFontFamily // 控件文本字体
horizontalAlignment: Text.AlignHCenter // 控件文本水平对齐方式
verticalAlignment: Text.AlignVCenter // 控件文本垂直对齐方式
wrapMode: Text.WrapAnywhere // 控件文本自动换行模式
clip: true // 控件文本超出边界时进行裁剪
function replaceBlank(str){ // 替换文本中的空格为换行符的方法
let newStr = str
newStr = newStr.replace(" ", "\n")
}
}
}
}
}
```
import QtQuick 2.0 import QtQuick.Controls 2.5 ListView { id: outerListView width: 200 height: 400 model: outerModel delegate: ItemDelegate { text: model.label width: outerListView.width ListView { id: innerListView width: 200 height: 300 model: model.children visible: expanded delegate: ItemDelegate { text: model.label width: innerListView.width highlight: checked ? Rectangle { color: "lightblue" } : null onClicked: { if (multiSelect) { checked = !checked; } else { outerListView.currentIndex = -1; checked = true; } } } } Rectangle { id: expandIcon anchors.right: parent.right anchors.verticalCenter: parent.verticalCenter width: 10 height: 10 color: "black" transform: expanded ? Rotation { origin.x: 5; origin.y: 5; angle: 90 } : Rotation { origin.x: 5; origin.y: 5; angle: 0 } MouseArea { anchors.fill: parent onClicked: expanded = !expanded } } property bool expanded: false property bool multiSelect: false property bool checked: false } } 横向LIstView
要实现横向的 `ListView`,可以在外层 `ListView` 的 `orientation` 属性中设置为 `ListView.Horizontal`。同时,需要将内层 `ListView` 的宽度设置为外层 `ListView` 中每个选项的宽度之和,以确保内层选项能够全部展示出来,可以通过外层 `ListView` 的 `contentWidth` 属性来获取。
下面是修改后的示例代码:
```qml
import QtQuick 2.0
import QtQuick.Controls 2.5
ListView {
id: outerListView
width: 400
height: 50
orientation: ListView.Horizontal
model: outerModel
delegate: ItemDelegate {
text: model.label
width: 100
ListView {
id: innerListView
width: outerListView.contentWidth
height: 50
model: model.children
visible: expanded
orientation: ListView.Horizontal
delegate: ItemDelegate {
text: model.label
width: 100
highlight: checked ? Rectangle { color: "lightblue" } : null
onClicked: {
if (multiSelect) {
checked = !checked;
} else {
outerListView.currentIndex = -1;
checked = true;
}
}
}
}
Rectangle {
id: expandIcon
anchors.right: parent.right
anchors.verticalCenter: parent.verticalCenter
width: 10
height: 10
color: "black"
transform: expanded ? Rotation { origin.x: 5; origin.y: 5; angle: 90 } : Rotation { origin.x: 5; origin.y: 5; angle: 0 }
MouseArea {
anchors.fill: parent
onClicked: expanded = !expanded
}
}
property bool expanded: false
property bool multiSelect: false
property bool checked: false
}
}
```
这样,就可以通过设置 `orientation` 属性将外层 `ListView` 设置为横向,同时将内层 `ListView` 的宽度设置为外层 `ListView` 中每个选项的宽度之和,来实现横向的 `ListView` 了。
相关推荐
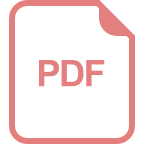
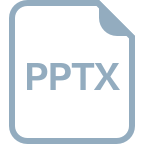













