import QtQuick 2.12 import QtQuick.Controls 2.2 import QtQuick.Dialogs 1.3 Item { width: 400 height: 400 // 模拟数据 ListModel { id: myModel ListElement { name: "张三"; age: 20 } ListElement { name: "李四"; age: 30 } ListElement { name: "王五"; age: 40 } } ListView { id: listView anchors.fill: parent model: DelegateModel { id: delegateModel model: myModel delegate: Rectangle { width: parent.width height: 50 color: ListView.isCurrentItem ? "lightblue" : "white" // 显示数据 Row { Text { text: name } Text { text: age } } // 点击弹出弹框 MouseArea { anchors.fill: parent onClicked: { myDialog.nameInput.text = name myDialog.ageInput.text = age myDialog.open() } } } } } // 弹框 Dialog { id: myDialog title: "编辑" width: 200 height: 150 visible: false Column { TextField { id: nameInput placeholderText: "姓名" } TextField { id: ageInput placeholderText: "年龄" } Row { Button { text: "保存" onClicked: { // 保存数据 delegateModel.items[listView.currentIndex].name = nameInput.text delegateModel.items[listView.currentIndex].age = ageInput.text myDialog.close() } } Button { text: "取消" onClicked: { myDialog.close() } } } } } }19行报错什么原因
时间: 2024-02-11 07:12:04 浏览: 35
根据代码和错误信息来看,错误信息是:
```
TypeError: Cannot read property 'items' of null
```
这个错误表示在第 19 行中,访问了一个空对象的 `items` 属性,因此导致了错误。具体来说,这个错误是因为在 `Button` 的 `onClicked` 中访问了 `delegateModel`,而 `delegateModel` 并没有被定义或者赋值。因此,需要在代码中将 `delegateModel` 定义或者赋值,以解决这个错误。具体的解决方法,可以根据实际情况来进行调整。
相关问题
Error: Qt.createQmlObject(): failed to create object:
如果在使用 `Qt.createQmlObject()` 方法时出现 `Error: Qt.createQmlObject(): failed to create object` 的错误,可能是因为你的 QML 代码存在语法错误或者逻辑错误。
可以尝试使用 `console.log()` 输出一些调试信息,帮助你找到错误的原因。比如在创建 `Process` 对象之前,可以输出一些信息,检查 import 是否正确,语法是否正确,如下所示:
```
var processQml = 'import QtQuick 2.0; import QtQuick.Window 2.0; import QtQuick.Dialogs 1.0; Process { command: "shutdown", arguments: ["/s", "/t", "0"] }'
console.log("processQml:", processQml)
var process = Qt.createQmlObject(processQml, parent)
console.log("process:", process)
process.start()
```
如果输出的信息中存在语法错误或者 import 错误,可以尝试修改 QML 代码,修复错误即可。如果确定 QML 代码没有问题,可以尝试将 `Qt.createQmlObject()` 方法的第二个参数 `parent` 改为 `null`,看看是否可以创建成功,代码如下:
```
var process = Qt.createQmlObject(processQml, null)
```
如果还是无法解决问题,可以将完整的 QML 代码和报错信息一起贴出来,我们可以一起来看看是哪里出了问题。
anchors.fill: parent onClicked: if(colorDialog == null){ colorDialog = Qt.createQmlObject("import QtQuick 2.2;import QtQuick.Dialogs 1.0; ColorDialog{}", colorPicker, "dynamic_color_dialog"); colorDialog.accepted.connect(colorPicker.onColorDialogAccepted); colorDialog.rejected.connect(colorPicker.onColorDialogRejected); colorDialog.open();
这段代码是在 `ColorPicker` 中定义的一个 `MouseArea` 元素,它将 `ColorPicker` 的大小设置为与其父元素相同,并在鼠标点击事件发生时打开一个颜色选择对话框。
`anchors.fill: parent` 属性用于将该元素的四个边缘锚定到其父元素的四个边缘,使其与父元素的大小相同。
`onClicked` 信号处理函数中,如果 `colorDialog` 对象不存在,则通过 `Qt.createQmlObject()` 动态创建一个 `ColorDialog` 对象,并将其父元素设置为 `colorPicker`。`colorDialog.accepted` 和 `colorDialog.rejected` 信号分别连接到 `ColorPicker` 中的 `onColorDialogAccepted()` 和 `onColorDialogRejected()` 槽函数中。最后,通过 `colorDialog.open()` 打开颜色选择对话框。
在 `ColorPicker` 中添加该元素后,当用户点击 `ColorPicker` 区域时,将会弹出一个颜色选择对话框,并且用户选择颜色后,会将选择的颜色设置到 `ColorPicker` 中。
注意,该代码中的 `ColorDialog` 是 Qt Quick Dialogs 中的一个组件,需要在 QML 文件中导入该组件才能使用。
相关推荐
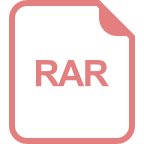
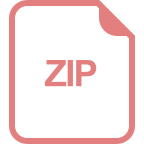
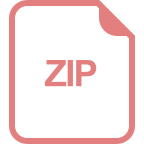












