联邦学习pytorch代码
时间: 2023-05-25 21:04:57 浏览: 341
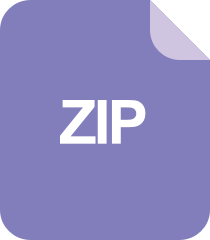
高分毕业设计-基于Pytorch分布式包的单机多核CPU实现横向联邦学习Python源码+文档说明+配置说明

联邦学习是一种新兴的分散式机器学习方法,它可以在保护个人隐私的前提下,使用分布式计算资源进行模型训练。下面是一个使用 PyTorch 实现的简单联邦学习示例代码:
首先,我们需要定义一个模型类:
```
import torch.nn as nn
import torch.optim as optim
class Net(nn.Module):
# define the layers of your model
def __init__(self):
super().__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
# define how data flows through the model
def forward(self, x):
x = self.fc1(x)
x = nn.functional.relu(x)
x = self.fc2(x)
x = nn.functional.sigmoid(x)
return x
```
接下来,我们需要为每个参与联邦学习的设备(例如智能手机、平板电脑等)定义一个自己本地的数据集。在本例中,我们假设每个设备的数据集都是由一些样本组成的数组。
```
# define the data partitions
data1 = torch.randn(100, 10).float()
target1 = torch.randint(0, 2, (100, 1)).float()
data2 = torch.randn(50, 10).float()
target2 = torch.randint(0, 2, (50, 1)).float()
data3 = torch.randn(50, 10).float()
target3 = torch.randint(0, 2, (50, 1)).float()
```
接下来,我们定义一个联邦学习过程的迭代函数。在每个迭代中,我们从所有参与方的本地数据集中随机选择一定数量的样本,将它们组合成一个全局数据集,并用这个全局数据集来训练模型。对于每个参与方,我们都要将训练得到的模型参数传回给他们,以便他们可以更新自己的本地模型。
```
def federated_train(model, devices, data, target, num_epochs=10, batch_size=10, lr=0.1):
# define the optimizer and loss function
optimizer = optim.SGD(model.parameters(), lr=lr)
criterion = nn.BCELoss()
# train the model on the given data
for epoch in range(num_epochs):
for device_idx, device in enumerate(devices):
# randomly partition the data
indices = torch.randperm(len(data[device_idx]))
batches = [indices[i:i+batch_size] for i in range(0, len(indices), batch_size)]
# train the model on each batch
for batch in batches:
optimizer.zero_grad()
inputs = data[device_idx][batch]
labels = target[device_idx][batch]
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
# send the updated model parameters back to the device
if device_idx < len(devices) - 1:
model_param = model.state_dict()
comm.send(model_param, dest=device_idx+1)
# receive the updated model parameters from the devices
for device_idx, device in enumerate(devices):
if device_idx > 0:
model_param = comm.recv(source=device_idx)
model.load_state_dict(model_param)
```
最后,我们将所有步骤组合在一起,并运行联邦学习:
```
# define the devices and data partitions
devices = [0, 1, 2]
data = [data1, data2, data3]
target = [target1, target2, target3]
# create the model and start federated training
model = Net()
federated_train(model, devices, data, target)
```
这是一个非常简单的例子,但可以作为一个入门级的基础,帮助你进一步了解联邦学习的实现方式。
阅读全文
相关推荐
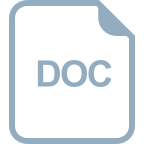
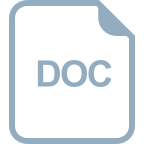
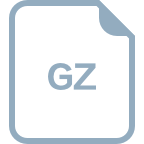





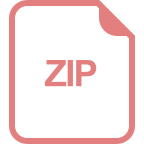
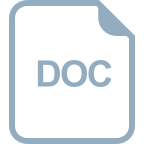






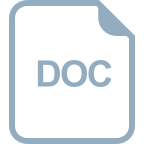
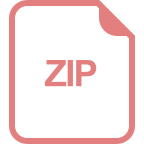