python 比较两个文件夹中的图片是否同名
时间: 2023-08-07 16:02:32 浏览: 272
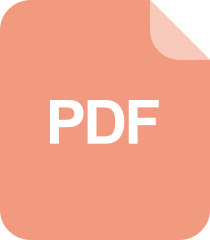
python模块导入的细节详解
可以使用 Python 的 os 模块和 glob 模块来比较两个文件夹中的图片是否同名。以下是一个示例代码:
```python
import os
import glob
folder1 = 'path/to/folder1'
folder2 = 'path/to/folder2'
# 获取两个文件夹中的图片文件名列表
files1 = [os.path.basename(f) for f in glob.glob(os.path.join(folder1, '*.jpg'))]
files2 = [os.path.basename(f) for f in glob.glob(os.path.join(folder2, '*.jpg'))]
# 比较两个列表中的文件名是否相同
for file1 in files1:
if file1 in files2:
print(f"{file1} exists in both folders.")
else:
print(f"{file1} doesn't exist in {folder2}.")
for file2 in files2:
if file2 not in files1:
print(f"{file2} doesn't exist in {folder1}.")
```
这段代码假设两个文件夹中都只包含 jpg 格式的图片文件。如果要比较其他格式的文件,可以修改 glob.glob() 中的参数。
阅读全文
相关推荐
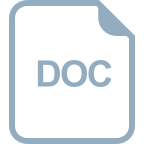
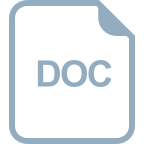

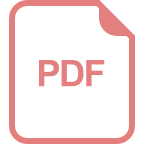







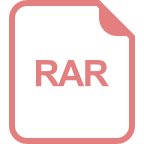
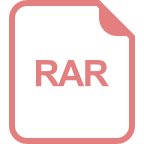




