strtok()函数分割字符串
时间: 2023-10-15 21:24:35 浏览: 105
strtok()函数是C语言中用于分割字符串的函数。它可以将一个字符串按照指定的分隔符进行拆分,并返回拆分后的子字符串。
函数原型如下:
```c
char *strtok(char *str, const char *delim);
```
参数说明:
- `str`:要进行分割的字符串。在第一次调用时传入需要分割的字符串,在后续调用时传入NULL。
- `delim`:分隔符字符串,可以包含多个分隔符。
函数返回值:
返回一个指向被分割出来的子字符串的指针,如果没有可分割的子字符串,返回NULL。
使用示例:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, world! This is a test.";
char *token;
token = strtok(str, " ,!"); // 使用空格、逗号和感叹号作为分隔符
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, " ,!"); // 继续下一个分隔符
}
return 0;
}
```
输出结果:
```
Hello
world
This
is
a
test
```
以上是`strtok()`函数的基本用法,你可以通过设置不同的分隔符来实现不同的字符串分割需求。
相关问题
如何使用strtok函数分割字符串?
在C语言中,可以使用strtok函数来分割字符串。strtok函数可以将一个字符串分割成多个子字符串,根据指定的分隔符进行分割。
下面是使用strtok函数分割字符串的示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello,World,How,Are,You";
char *token;
// 使用逗号作为分隔符
token = strtok(str, ",");
// 循环获取分割后的子字符串
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, ",");
}
return 0;
}
```
运行上述代码,输出结果为:
```
Hello
World
How
Are
You
```
在C++中,可以使用stringstream类来分割字符串。下面是使用stringstream类分割字符串的示例代码:
```cpp
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string str = "Hello,World,How,Are,You";
std::stringstream ss(str);
std::string token;
// 使用逗号作为分隔符
while (std::getline(ss, token, ',')) {
std::cout << token << std::endl;
}
return 0;
}
```
运行上述代码,输出结果为:
```
Hello
World
How
Are
You
```
Matlab中如何用strtok 函数取出字符串中的第10到14个元素
在MATLAB中,`strtok`函数主要用于将一个字符串按照指定的分隔符拆分成多个子串,但它本身并不直接支持按索引提取特定位置的子串。如果你想要获取字符串中的第10到14个字符(注意MATLAB中的索引从1开始),你需要先确定分隔符并使用循环或其他方法来获取这些片段。
这里假设你想从无分隔符的纯文本字符串中提取部分字符,你可以这样做:
```matlab
% 假设原字符串名为 'myString'
originalStr = 'myStringToExtract';
% 如果字符串长度小于15,就无法提取10到14个字符
if length(originalStr) < 15
disp('字符串太短,无法提取10到14个字符');
else
% 提取第10到14个字符(包括第10和第14个)
extractedChars = originalStr(10:14);
disp(extractedChars);
end
```
如果你想按照某个特定的分隔符如空格、逗号等来提取,则需要先用`strtok`分割,然后遍历结果。例如,如果分隔符是空格:
```matlab
delimiter = ' ';
tokens = strtok(originalStr, delimiter);
% 获取第10到14个token(每个token对应一个字符串片段)
indexStart = 9; % 因为从0开始计数,所以10号对应的是第9个元素
indexEnd = 13;
selectedTokens = tokens(indexStart:indexEnd);
disp(selectedTokens);
```
请注意,在实际操作前,你需要检查原始字符串的确切内容和长度,以避免潜在的索引越界问题。
阅读全文
相关推荐
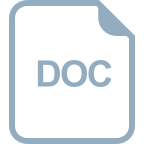
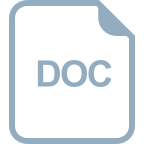
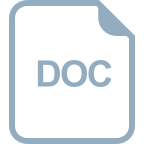
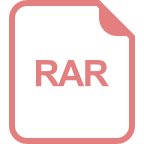





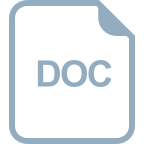
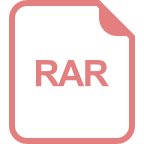
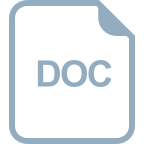




