clf = gcForest(shape_1X=(1, 3), window=[2])举例说明
时间: 2023-06-02 22:05:25 浏览: 55
这是一个使用gcForest算法构建分类器的代码示例。gcForest是一种基于随机森林的深度森林算法,具有较高的准确性和效率。该代码中的shape_1X和window参数用于定义输入数据的形状和滑动窗口的大小。具体实现可以参考相关文档和代码库。
相关问题
tree_clf = DecisionTreeClassifier(max_depth=2)
这是一个使用最大深度为2的决策树分类器的示例。决策树是一种基于树形结构的监督学习算法,它可以用于分类和回归问题。在这个示例中,我们使用的是sklearn库中的DecisionTreeClassifier类。max_depth参数指定了决策树最大深度,也就是树的层数。这个参数的值越大,模型就越复杂,可能会导致过拟合。如果max_depth设置得太小,模型就会欠拟合。因此,需要根据实际情况进行调整。
import pandas as pd import numpy as np from sklearn.datasets import load_digits from sklearn.metrics import * from sklearn.cluster import * digits = load_digits() digits_X = digits.data digits_y = digits.target n_clusters = 10 clf =(n_clusters=n_clusters, random_state=0) clf.load_digits() result = homogeneity_completeness_v_measure(digits_y, clf.labels_)
在你提供的代码中,有一些问题需要修改才能正确运行。
首先,你在定义 `clf` 时缺少了 `KMeans` 这个类的名字,应该改为:
```python
clf = KMeans(n_clusters=n_clusters, random_state=0)
```
接着,你需要用 `clf.fit()` 方法来拟合模型,而不是 `clf.load_digits()`。
最后,你可以使用 `homogeneity_completeness_v_measure()` 方法来计算聚类结果的完整性、同质性和V-measure值。需要注意的是,这个方法需要传入真实标签和预测标签两个参数,因此我们需要用 `clf.predict(digits_X)` 来获取预测标签。修改后的代码如下:
```python
import pandas as pd
import numpy as np
from sklearn.datasets import load_digits
from sklearn.metrics import *
from sklearn.cluster import *
digits = load_digits()
digits_X = digits.data
digits_y = digits.target
n_clusters = 10
clf = KMeans(n_clusters=n_clusters, random_state=0)
clf.fit(digits_X)
result = homogeneity_completeness_v_measure(digits_y, clf.predict(digits_X))
print("Homogeneity: {:.3f}, Completeness: {:.3f}, V-measure: {:.3f}".format(result[0], result[1], result[2]))
```
希望这个回答对你有所帮助!
相关推荐
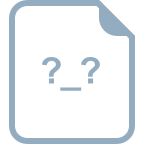












