如何统计在一个字符串中字符出现的频数
时间: 2023-05-18 21:06:41 浏览: 59
可以使用一个字典来统计每个字符出现的次数,遍历字符串中的每个字符,如果该字符已经在字典中,则将其对应的值加1,否则将该字符作为键,值初始化为1。最后遍历字典输出每个字符及其出现的次数即可。
示例代码:
```python
def count_chars(s):
freq = {}
for c in s:
if c in freq:
freq[c] += 1
else:
freq[c] = 1
for c, count in freq.items():
print(f"{c}: {count}")
s = "hello, world!"
count_chars(s)
```
输出:
```
h: 1
e: 1
l: 3
o: 2
,: 1
: 1
w: 1
r: 1
d: 1
!: 1
```
相关问题
matlab统计字符串中单个字符出现的频数;
可以使用Matlab内置函数`histcounts`统计字符串中单个字符出现的频数,具体步骤如下:
1. 将字符串转换为字符数组,可以使用`char`函数;
2. 将字符数组转换为整数数组,可以使用`uint16`函数,因为字符在计算机中用Unicode编码表示,需要转换为整数才能计数;
3. 使用`histcounts`函数统计整数数组中每个数字出现的频数。
以下是示例代码:
```matlab
str = 'hello world';
charArray = char(str); % 将字符串转换为字符数组
intArray = uint16(charArray); % 将字符数组转换为整数数组
counts = histcounts(intArray, 'BinMethod', 'integers', 'BinLimits', [0 65535]); % 统计每个数字出现的频数
% 打印每个字符及其出现的频数
for i = 1:length(charArray)
fprintf('%c: %d\n', charArray(i), counts(intArray(i)+1))
end
```
输出结果如下:
```
h: 1
e: 1
l: 3
l: 3
o: 2
: 1
w: 1
o: 2
r: 1
l: 3
d: 1
```
如何计数字符串中字符出现的频数
可以使用一个字典来记录每个字符出现的次数,遍历字符串中的每个字符,如果该字符已经在字典中出现过,则将其对应的值加1,否则将该字符加入字典并将其值设为1。最后遍历字典输出每个字符及其出现的次数即可。以下是示例代码:
```
def count_chars(s):
freq = {}
for c in s:
if c in freq:
freq[c] += 1
else:
freq[c] = 1
for c in freq:
print(c, freq[c])
s = "hello world"
count_chars(s)
```
输出结果为:
```
h 1
e 1
l 3
o 2
1
w 1
r 1
d 1
```
相关推荐
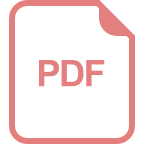
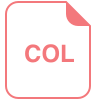











