三周加速度计计算角度的C语言代码
时间: 2023-07-15 21:15:07 浏览: 47
以下是一个简单的三周加速度计计算角度的C语言代码:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265
int main() {
// 初始化参数,x、y、z轴的加速度值
float ax = 0.0, ay = 0.0, az = 0.0;
// 初始化变量,用于保存角度
float pitch = 0.0, roll = 0.0;
// 读取加速度计数据
scanf("%f %f %f", &ax, &ay, &az);
// 计算pitch角度
pitch = atan2(ax, sqrt(ay*ay + az*az)) * 180.0 / PI;
// 计算roll角度
roll = atan2(ay, sqrt(ax*ax + az*az)) * 180.0 / PI;
// 输出结果
printf("pitch = %.2f degrees, roll = %.2f degrees\n", pitch, roll);
return 0;
}
```
这段代码使用了数学库中的 `atan2` 函数来计算角度。其中 `atan2(y, x)` 的返回值表示点(x, y)与原点的连线与x轴正方向的夹角,单位为弧度。最后将弧度转换为角度并输出。需要注意的是,这里假设加速度计的测量值已经经过预处理,包括去除重力加速度等因素。
相关问题
三周加速度计计算倾斜角度的C语言代码
以下是一个简单的三周加速度计计算倾斜角度的C语言代码:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
int main()
{
float ax, ay, az; // 三轴加速度数据
float roll, pitch; // 倾斜角度
// 读取三轴加速度数据
printf("请输入三轴加速度数据(单位:m/s^2):\n");
printf("ax = ");
scanf("%f", &ax);
printf("ay = ");
scanf("%f", &ay);
printf("az = ");
scanf("%f", &az);
// 计算倾斜角度
roll = atan2(ay, sqrt(ax*ax + az*az)) * 180.0 / PI;
pitch = atan2(-ax, sqrt(ay*ay + az*az)) * 180.0 / PI;
// 输出倾斜角度
printf("倾斜角度为:\n");
printf("roll = %.2f°\n", roll);
printf("pitch = %.2f°\n", pitch);
return 0;
}
```
该代码使用了三周加速度计的三轴加速度数据,通过计算得到倾斜角度。其中,使用了 atan2 函数来计算反正切值,然后将弧度转换为角度。注意,该代码中的角度单位为度。
只使用Z轴的加速度值计算角度的C语言代码
以下是只使用Z轴加速度值计算角度的C语言代码:
```c
#include <stdio.h>
#include <math.h>
int main()
{
float acc_z, angle;
printf("Enter Z-axis acceleration value: ");
scanf("%f", &acc_z);
angle = atan(acc_z / 9.81) * (180/M_PI); // 9.81 is the acceleration due to gravity in m/s^2
printf("Angle = %f degrees\n", angle);
return 0;
}
```
该代码通过读取用户输入的Z轴加速度值,使用反正切函数计算出角度,并将结果以度数形式输出。需要注意的是,使用只有单一轴加速度值来计算角度并不完全准确,因为这种方法无法考虑到设备的旋转和倾斜等因素。
相关推荐
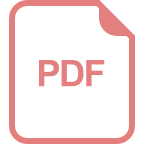












