python 如何实现dfs
时间: 2023-03-14 17:02:55 浏览: 88
Python 可以使用递归函数来实现深度优先搜索(DFS)算法。它将搜索树分解为两个部分:“发现”过程和“探索”过程。“发现”过程会找到新的可能有用的节点,而“探索”过程会递归地去搜索这些新发现的节点,以达到最终目标。
相关问题
用python实现dfs遍历
可以使用以下Python代码实现深度优先搜索(DFS)遍历:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
dfs算法python实现
下面是DFS算法的Python实现示例:
```python
def dfs(graph, start, visited=None):
if visited is None:
visited = set() # 创建一个空集合存储访问过的节点
visited.add(start) # 将起点加入到访问集合中
print(start) # 打印当前节点
for next_node in graph[start] - visited: # 遍历当前节点的邻接节点
dfs(graph, next_node, visited) # 对未访问的邻接节点进行深度优先搜索
return visited
```
其中,`graph`表示图的邻接表,`start`表示起点。在函数中,我们首先将起点加入到访问集合中,然后打印当前节点,接着遍历当前节点的邻接节点,对未访问的邻接节点进行深度优先搜索。最终,返回访问过的节点集合。
相关推荐
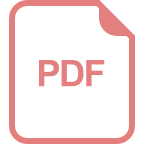
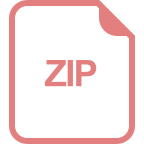
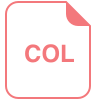
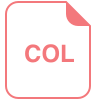
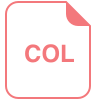
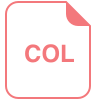
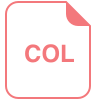







