请帮我用mworks写一个插值相关的代码
时间: 2024-10-27 17:05:19 浏览: 57
MWorks虽然是一款UI/UX设计工具,并非直接用于编写程序代码,尤其是数学计算如插值算法的部分。不过,如果你要在MWorks项目中集成一个插值功能,通常会使用Objective-C或Swift这样的编程语言,在其内置的编辑器或外部IDE中完成。
假设你想要使用Objective-C来实现线性插值,你可以这样做:
```objective-c
// 导入数学库
#import <Foundation/Foundation.h>
// 线性插值函数示例
double linearInterpolation(double x, double y0, double y1, double x0, double x1) {
return (x - x0) * (y1 - y0) / (x1 - x0) + y0;
}
// 使用方法
double inputValue = ...; // 输入点的值
double outputValue = linearInterpolation(inputValue, startValue, endValue, startPoint, endPoint);
```
在MWorks中,你需要将这个代码块复制到对应的Objective-C或Swift文件里,然后在需要的地方调用`linearInterpolation`方法。
相关问题
用mworks写时域采样与频域采样的试验代码
在MWorks中编写时域采样与频域采样的试验代码通常涉及到信号处理模块的使用。以下是一个简化的示例:
**时域采样 (Sample in Time Domain)**:
```c++
#include "MWCore.h"
MWReal-time Signal signal;
void Initialize() {
// 创建一个简单的正弦波信号
signal = CreateSineWave(100, 1); // 频率100Hz, 幅度1
// 每毫秒采样一次
SetSampleRate(SampleEveryMillisecond);
}
void Step() {
MWReal sample = GetNextSample(signal); // 获取当前时间点的样本值
Print(sample); // 打印到控制台
}
void Terminate() {
DestroySignal(signal);
}
```
**频域采样 (Sample in Frequency Domain)**:
```c++
#include "MWMath.h"
void Initialize() {
// 同样创建一个正弦波信号
MWReal-time Signal signal = CreateSineWave(100, 1);
// 进行快速傅里叶变换 (FFT)
MWComplex spectrum[] = FFT(signal, SampleRate());
}
void Step() {
// 这里假设已经计算了频谱
for (int i = 0; i < NumSpectrumBins; i++) {
MWComplex bin = Spectrum[i];
Print(bin.Real + "j" + bin.Imaginary); // 打印复数形式的频率分量
}
}
void Terminate() {
DestroySignal(signal);
}
```
注意:实际应用中你需要替换`Print`函数为适合你的数据存储和可视化的函数。
MWORKS编写Kalman filter程序 帮我写代码
MWorks是一款用于实时嵌入式系统的软件平台,它支持多种编程语言,包括C++。在MWorks中编写卡尔曼滤波(Kalman Filter)的程序,你需要先了解基本的卡尔曼滤波理论,然后使用MWorks的数据类型和API。
下面是一个简化的C++代码示例,演示如何在MWorks中创建一个线性卡尔曼滤波器的基本结构:
```cpp
#include <MWKf.h> // 引入MWorks的卡尔曼滤波库
class MyFilter : public MWKF::Linear {
public:
MyFilter(double dt) : MWKF::Linear(dt) {
// 初始化状态矩阵 A (系统动态模型), 状态协方差矩阵 P, 控制矩阵 B,
// 测量矩阵 H, 测量噪声协方差 R, 和过程噪声协方差 Q
A = ...; // 系统动力学矩阵
B = ...; // 控制矩阵
H = ...; // 测量矩阵
R = ...; // 测量噪声协方差
Q = ...; // 过程噪声协方差
}
// ... 其他必要的函数,如预测(predict)、更新(update)等
};
// 使用示例
MyFilter kf(0.1); // dt 是采样周期
kf.predict(); // 预测下一个时刻的状态
kf.update(measurement); // 更新状态,measurement是你接收到的新测量值
```
请将...部分替换为你实际的矩阵值,并确保它们符合你的系统模型。记得MWorks可能会有自己的API和数据结构,所以请查阅其官方文档或参考案例来完成编码。
阅读全文
相关推荐
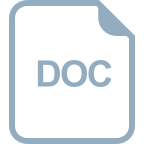
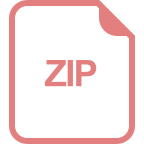













