给出一段java,获取InputStream获取前10个字节的文件头判断文件的类型,再保存文件
时间: 2024-09-30 12:02:12 浏览: 21
在Java中,获取InputStream的前10个字节作为文件头并判断文件类型后保存整个文件,通常涉及到几个步骤。首先,你需要创建一个BufferedReader来逐字节读取,然后分析这10个字节来确定文件类型。这里是一个简化的示例:
```java
import java.io.*;
public class FileHeaderTypeDeterminer {
public static void main(String[] args) {
try {
String filePath = "path_to_your_file"; // 替换为实际文件路径
byte[] headerBytes = new byte[10];
// 读取文件头
FileInputStream fis = new FileInputStream(filePath);
fis.read(headerBytes);
fis.close(); // 关闭输入流以释放资源
// 判断文件类型
String fileType = determineFileType(headerBytes);
System.out.println("File type is: " + fileType);
// 分析文件类型后决定如何保存,例如,如果是图片,则另存为原格式;如果是文本,可以保持不变等
saveFileBasedOnType(filePath, fileType);
} catch (IOException e) {
e.printStackTrace();
}
}
private static String determineFileType(byte[] headerBytes) {
// 实现具体的文件类型判断逻辑,如查看第一个字符可能是.txt、.jpg、.pdf等
// 这里只是一个简化示例,真实场景下可能需要更复杂的解析逻辑
if (headerBytes[0] == 'M' && headerBytes[1] == 'J' && headerBytes[2] == 'P') { // 举例JPEG
return "image/jpeg";
} else if (Arrays.copyOfRange(headerBytes, 0, 4).equals(new byte[]{0x4D, 0x5A})) { // 举例Windows PE
return "application/octet-stream";
}
// 添加更多类型的判断...
throw new IllegalArgumentException("Unsupported file format");
}
private static void saveFileBasedOnType(String filePath, String fileType) throws IOException {
switch (fileType) {
case "image/jpeg":
saveAsJPEG(filePath, filePath + ".jpg"); // 保存为新的jpeg格式
break;
// 其他类型的处理逻辑...
default:
copyFile(filePath, filePath); // 如果无法确定,简单地复制文件
}
}
private static void copyFile(String srcPath, String dstPath) throws IOException {
InputStream in = new FileInputStream(srcPath);
OutputStream out = new FileOutputStream(dstPath);
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) != -1) {
out.write(buffer, 0, length);
}
in.close();
out.close();
}
// 保存为特定格式的方法,这里只演示了JPEG,其他格式可根据需求添加
private static void saveAsJPEG(String srcPath, String dstPath) throws IOException {
FileInputStream fis = new FileInputStream(srcPath);
BufferedImage image = ImageIO.read(fis);
File dest = new File(dstPath);
ImageIO.write(image, "jpg", dest);
fis.close();
}
}
```
**相关问题--:**
1. 如果文件类型复杂,如何更准确地判断?
2. 如何处理文件类型未知的情况?
3. 在保存文件时如果需要考虑编码问题怎么办?
相关推荐
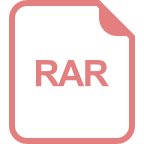
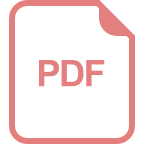
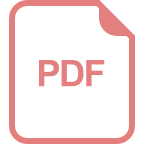
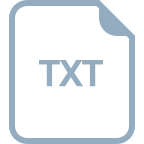
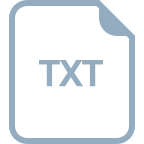
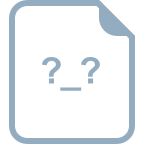
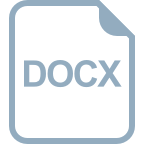
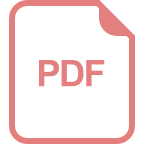
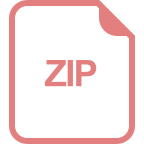
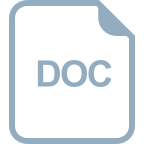
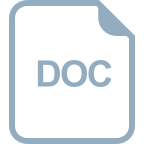
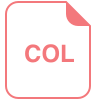
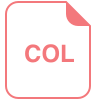
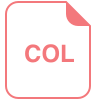
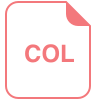
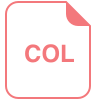

