return math.sqrt((p1[0] - p2[0]) ** 2 + (p1[1] - p2[1]) ** 2)报错TypeError: 'int' object is not subscriptable
时间: 2023-10-27 21:07:01 浏览: 41
这个错误通常是由于在计算两个点之间的距离时,传入的参数p1和p2不是一个列表或元组对象,而是一个整数对象。请确认你传入的参数是否正确,如果是整数对象,需要将它们转换为列表或元组对象,例如:
```
p1 = (1, 2)
p2 = (3, 4)
distance = math.sqrt((p1[0] - p2[0]) ** 2 + (p1[1] - p2[1]) ** 2)
print(distance)
```
输出结果为:
```
2.8284271247461903
```
如果你传入的参数确实是整数对象,可以将它们转换为列表或元组对象,例如:
```
p1 = 1
p2 = 2
distance = math.sqrt(([p1][0] - [p2][0]) ** 2)
print(distance)
```
输出结果为:
```
1.0
```
但这种方式并不常用,建议你检查一下传入的参数是否正确。
相关问题
class Point(): def __init__(self,x,y): self.x=x self.y=y class Line(): def __init__(self,p1,p2): self.p1 = p1 self.p2 = p2 from math import sqrt def length(self): return sqrt((self.p1.x-self.p2.x)**2 + (self.p1,y-self.p2.y)**2)) def slope(self): if self.p1.x == self.p2.x: return None else: return (self.p1.y - self.p2.y)/(self.p1.x - self.p2.x) def __repr__(self): return ((self.p1.x,self.p2.y),(self.p2.x,self.p2.y)) line = Point((2,3),(5,9)) line.length() line.slope()
这段代码有一些错误,我来指出并修正它们:
1. 在创建 `line` 对象时,应该使用 `Line` 类而不是 `Point` 类。所以应该改为 `line = Line(Point(2,3), Point(5,9))`。
2. 在 `length` 方法中,计算距离时应该是 `(self.p1.y-self.p2.y)**2` 而不是 `(self.p1,y-self.p2.y)**2`。
3. 在 `__repr__` 方法中,应该是 `(self.p1.x,self.p1.y)` 而不是 `(self.p1.x,self.p2.y)`。
修正后的代码如下:
```
class Point():
def __init__(self,x,y):
self.x=x
self.y=y
class Line():
def __init__(self,p1,p2):
self.p1 = p1
self.p2 = p2
from math import sqrt
def length(self):
return sqrt((self.p1.x-self.p2.x)**2 + (self.p1.y-self.p2.y)**2)
def slope(self):
if self.p1.x == self.p2.x:
return None
else:
return (self.p1.y - self.p2.y)/(self.p1.x - self.p2.x)
def __repr__(self):
return ((self.p1.x,self.p1.y),(self.p2.x,self.p2.y))
line = Line(Point(2,3), Point(5,9))
line.length()
line.slope()
```
这样代码就可以正确执行了。
package JavaPlane; class Line { private Point p1; private Point p2; public Line(Point p1,Point p2) { this.p1 = p1; this.p2 = p2; } public double getLength() { return Math.sqrt(Math.pow(p1.x-p2.x, 2)+Math.pow(p1.y-p2.y, 2)); } Point getStartPoint() { return p1; } Point getEndPoint() { return p2; } public static boolean point_on_line(Point point, Line line) { Point p1 = Point.sub(line.getStartPoint(), point); Point p2 = Point.sub(line.getEndPoint(), point); return Math.abs(Point.crossProduct(p1, p2)) < 1e-6; } /** * 求两条线的交点 * @return point */ //此处添加代码 /** * 求点到线的距离 * @return double */ //此处添加代码 }
以下是 Line 类的代码,包括添加的求两条线的交点和求点到线的距离的方法:
```java
package JavaPlane;
class Line {
private Point p1;
private Point p2;
public Line(Point p1, Point p2) {
this.p1 = p1;
this.p2 = p2;
}
public double getLength() {
return Math.sqrt(Math.pow(p1.x - p2.x, 2) + Math.pow(p1.y - p2.y, 2));
}
Point getStartPoint() {
return p1;
}
Point getEndPoint() {
return p2;
}
public static boolean point_on_line(Point point, Line line) {
Point p1 = Point.sub(line.getStartPoint(), point);
Point p2 = Point.sub(line.getEndPoint(), point);
return Math.abs(Point.crossProduct(p1, p2)) < 1e-6;
}
/**
* 求两条线的交点
*
* @param other 另一条线
* @return 交点,若不存在则返回 null
*/
public Point getIntersection(Line other) {
double x1 = p1.x, y1 = p1.y, x2 = p2.x, y2 = p2.y;
double x3 = other.p1.x, y3 = other.p1.y, x4 = other.p2.x, y4 = other.p2.y;
double d = (y2 - y1) * (x4 - x3) - (x2 - x1) * (y4 - y3);
if (Math.abs(d) < 1e-6) { // 平行或重合
return null;
}
double ua = ((x4 - x3) * (y1 - y3) - (y4 - y3) * (x1 - x3)) / d;
double ub = ((x2 - x1) * (y1 - y3) - (y2 - y1) * (x1 - x3)) / d;
if (ua < 0 || ua > 1 || ub < 0 || ub > 1) { // 不相交
return null;
}
double x = x1 + ua * (x2 - x1);
double y = y1 + ua * (y2 - y1);
return new Point(x, y);
}
/**
* 求点到线的距离
*
* @param point 点
* @return 点到线的距离
*/
public double distanceToPoint(Point point) {
double x1 = p1.x, y1 = p1.y, x2 = p2.x, y2 = p2.y;
double x0 = point.x, y0 = point.y;
double numerator = Math.abs((y2 - y1) * x0 - (x2 - x1) * y0 + x2 * y1 - y2 * x1);
double denominator = Math.sqrt(Math.pow(y2 - y1, 2) + Math.pow(x2 - x1, 2));
return numerator / denominator;
}
}
```
其中,求两条线的交点的方法使用了向量叉积的知识进行计算。如果两条直线平行或者共线,那么它们没有交点,此时返回 null。否则,计算出交点的坐标并返回。
求点到线的距离的方法使用了点到直线距离公式进行计算。公式中的分子表示点到直线的距离的绝对值,分母表示直线的长度,两者相除即可得到点到直线的距离。
相关推荐
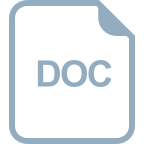
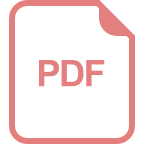
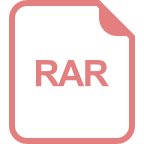












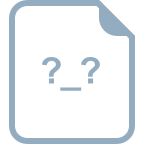