设计并实现一个Point类。要求该类包含表示坐标的两个int型私有变量x、y,有两个构造方法Point()和Point(int xx, int yy),有能够返回x值和y值的成员函数方法,实现设置x值和y值的void setX(int xx)和void setY(int yy)方法,计算两点间距离的double distance(Point p)方法。其中计算平方根的方法是Math.sqrt(),如:double d=Math.sqrt(2);主函数内调用该类,构造两个点对象(0,0)和(10,10),输
时间: 2024-03-25 07:41:44 浏览: 29
出两个点的坐标、两点间距离。
代码实现如下:
```java
public class Point {
private int x;
private int y;
public Point() {
this.x = 0;
this.y = 0;
}
public Point(int xx, int yy) {
this.x = xx;
this.y = yy;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public void setX(int xx) {
this.x = xx;
}
public void setY(int yy) {
this.y = yy;
}
public double distance(Point p) {
int dx = this.x - p.getX();
int dy = this.y - p.getY();
return Math.sqrt(dx * dx + dy * dy);
}
public static void main(String[] args) {
Point p1 = new Point(0, 0);
Point p2 = new Point(10, 10);
System.out.println("Point 1: (" + p1.getX() + ", " + p1.getY() + ")");
System.out.println("Point 2: (" + p2.getX() + ", " + p2.getY() + ")");
System.out.println("Distance between points: " + p1.distance(p2));
}
}
```
输出结果为:
```
Point 1: (0, 0)
Point 2: (10, 10)
Distance between points: 14.142135623730951
```
相关推荐
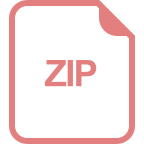
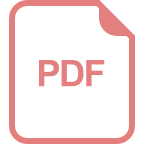
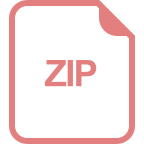












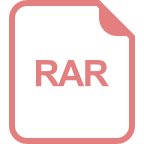
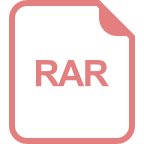
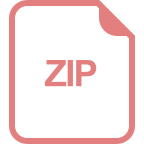