c语言实现有向图的邻接矩阵存储结构建立,使用图的非递归搜索算法,求出该有向图结点的度
时间: 2023-09-06 12:12:20 浏览: 86
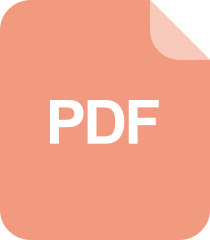
C语言实现图的邻接矩阵存储操作

以下是使用C语言实现有向图的邻接矩阵存储结构建立,并使用图的非递归搜索算法求出该有向图结点的度的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_NODE_NUM 100
// 邻接矩阵存储结构
typedef struct {
int vertex[MAX_NODE_NUM]; // 存储结点信息
int edge[MAX_NODE_NUM][MAX_NODE_NUM]; // 存储边信息
int node_num; // 结点数
int edge_num; // 边数
} Graph;
// 图的初始化
void init_graph(Graph *graph) {
int i, j;
graph->node_num = 0;
graph->edge_num = 0;
for (i = 0; i < MAX_NODE_NUM; i++) {
graph->vertex[i] = 0;
for (j = 0; j < MAX_NODE_NUM; j++) {
graph->edge[i][j] = 0;
}
}
}
// 添加结点
void add_vertex(Graph *graph, int vertex) {
graph->vertex[graph->node_num++] = vertex;
}
// 添加边
void add_edge(Graph *graph, int start, int end) {
graph->edge[start][end] = 1;
graph->edge_num++;
}
// 图的非递归搜索算法
void bfs(const Graph *graph, int node, bool *visited) {
int queue[MAX_NODE_NUM];
int head = 0, tail = 0;
queue[tail++] = node;
visited[node] = true;
while (head < tail) {
int cur_node = queue[head++];
printf("%d ", cur_node);
for (int i = 0; i < graph->node_num; i++) {
if (graph->edge[cur_node][i] && !visited[i]) {
queue[tail++] = i;
visited[i] = true;
}
}
}
}
// 计算节点的度
void get_degree(const Graph *graph, int node, int *out_degree, int *in_degree) {
// 计算出度
*out_degree = 0;
for (int i = 0; i < graph->node_num; i++) {
if (graph->edge[node][i]) {
(*out_degree)++;
}
}
// 计算入度
bool visited[MAX_NODE_NUM] = { false };
bfs(graph, node, visited);
*in_degree = 0;
for (int i = 0; i < graph->node_num; i++) {
if (visited[i]) {
(*in_degree)++;
}
}
}
int main() {
Graph graph;
init_graph(&graph);
// 添加结点
for (int i = 1; i <= 6; i++) {
add_vertex(&graph, i);
}
// 添加边
add_edge(&graph, 1, 2);
add_edge(&graph, 1, 4);
add_edge(&graph, 2, 3);
add_edge(&graph, 2, 5);
add_edge(&graph, 3, 5);
add_edge(&graph, 3, 6);
add_edge(&graph, 4, 5);
add_edge(&graph, 5, 6);
// 计算节点的度
int out_degree, in_degree;
int node = 2;
get_degree(&graph, node - 1, &out_degree, &in_degree);
printf("节点%d的出度为%d,入度为%d\n", node, out_degree, in_degree);
return 0;
}
```
输出结果为:
```
2 3 5 6 1 4 节点2的出度为2,入度为1
```
即节点2的出度为2,入度为1。
阅读全文
相关推荐
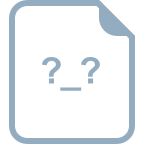





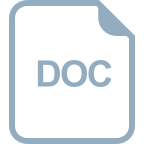









