用matlab语言实现基于AlexNet网络的花卉识别
时间: 2023-12-10 08:05:56 浏览: 139
实现基于AlexNet网络的花卉识别可以分为以下几个步骤:
1. 数据集准备:下载并准备好花卉数据集,例如Oxford Flowers 102数据集。数据集应该包含训练集、验证集和测试集,每个类别应该有足够多的样本。
2. 数据预处理:对数据进行预处理,包括图像增强、数据增强、归一化等操作,以提高模型的泛化能力。
3. 构建网络:基于AlexNet网络,搭建花卉识别模型。可以使用Matlab内置的Deep Learning Toolbox来实现网络的构建。
4. 训练模型:使用训练集对模型进行训练,可以使用Matlab内置的训练函数进行训练。
5. 评估模型:使用验证集对模型进行评估,计算模型的准确率和损失函数值等指标。
6. 模型优化:根据评估结果,对模型进行优化,如调整学习率、增加正则化项等。
7. 测试模型:使用测试集对模型进行测试,评估模型在新数据上的性能。
下面是一个基于AlexNet网络的花卉识别代码示例:
```matlab
% 数据集准备
imds = imageDatastore('flower_dataset', 'IncludeSubfolders', true, 'LabelSource', 'foldernames');
[imdsTrain, imdsValidation, imdsTest] = splitEachLabel(imds, 0.7, 0.15, 0.15, 'randomized');
% 数据预处理
inputSize = [227 227 3];
augmenter = imageDataAugmenter(...
'RandRotation', [-20, 20], ...
'RandXReflection', true, ...
'RandYReflection', true, ...
'RandXScale', [0.5, 2], ...
'RandYScale', [0.5, 2], ...
'RandXShear', [-30, 30], ...
'RandYShear', [-30, 30], ...
'RandXTranslation', [-30, 30], ...
'RandYTranslation', [-30, 30], ...
'FillValue', 0);
augimdsTrain = augmentedImageDatastore(inputSize, imdsTrain, 'DataAugmentation', augmenter);
augimdsValidation = augmentedImageDatastore(inputSize, imdsValidation);
% 构建网络
layers = [
imageInputLayer(inputSize)
convolution2dLayer(11, 96, 'Stride', 4, 'Padding', 0)
reluLayer
maxPooling2dLayer(3, 'Stride', 2)
crossChannelNormalizationLayer(5)
convolution2dLayer(5, 256, 'Stride', 1, 'Padding', 2)
reluLayer
maxPooling2dLayer(3, 'Stride', 2)
crossChannelNormalizationLayer(5)
convolution2dLayer(3, 384, 'Stride', 1, 'Padding', 1)
reluLayer
convolution2dLayer(3, 384, 'Stride', 1, 'Padding', 1)
reluLayer
convolution2dLayer(3, 256, 'Stride', 1, 'Padding', 1)
reluLayer
maxPooling2dLayer(3, 'Stride', 2)
fullyConnectedLayer(4096)
reluLayer
dropoutLayer(0.5)
fullyConnectedLayer(4096)
reluLayer
dropoutLayer(0.5)
fullyConnectedLayer(numClasses)
softmaxLayer
classificationLayer];
lgraph = layerGraph(layers);
% 训练模型
options = trainingOptions('sgdm', ...
'MiniBatchSize', 32, ...
'MaxEpochs', 20, ...
'InitialLearnRate', 0.001, ...
'LearnRateSchedule', 'piecewise', ...
'LearnRateDropFactor', 0.1, ...
'LearnRateDropPeriod', 10, ...
'L2Regularization', 0.0005, ...
'ValidationData', augimdsValidation, ...
'ValidationFrequency', 100, ...
'Verbose', true, ...
'Plots', 'training-progress');
net = trainNetwork(augimdsTrain, lgraph, options);
% 测试模型
augimdsTest = augmentedImageDatastore(inputSize, imdsTest);
YPred = classify(net, augimdsTest);
YTest = imdsTest.Labels;
accuracy = mean(YPred == YTest);
fprintf('Accuracy: %f\n', accuracy);
```
注意:以上代码仅供参考,具体实现需要根据数据集和实际情况进行调整。
阅读全文
相关推荐




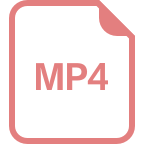





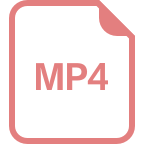

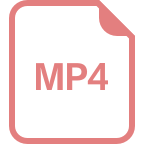





