用Python写一个简单的贪吃蛇游戏
时间: 2023-03-26 07:02:04 浏览: 85
非常感谢您的提问,我可以回答这个问题。以下是一个简单的 Python 贪吃蛇游戏的代码示例:
```python
import pygame
import random
# 游戏窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
# 贪吃蛇方块大小
BLOCK_SIZE = 20
# 颜色定义
BLACK = (, , )
WHITE = (255, 255, 255)
GREEN = (, 255, )
RED = (255, , )
# 初始化 Pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('贪吃蛇')
# 创建时钟对象
clock = pygame.time.Clock()
# 定义贪吃蛇类
class Snake:
def __init__(self):
self.body = [(4, 1), (3, 1), (2, 1), (1, 1)]
self.direction = 'right'
def move(self):
head = self.body[]
if self.direction == 'right':
new_head = (head[] + 1, head[1])
elif self.direction == 'left':
new_head = (head[] - 1, head[1])
elif self.direction == 'up':
new_head = (head[], head[1] - 1)
elif self.direction == 'down':
new_head = (head[], head[1] + 1)
self.body.insert(, new_head)
self.body.pop()
def draw(self):
for block in self.body:
rect = pygame.Rect(block[] * BLOCK_SIZE, block[1] * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
pygame.draw.rect(window, GREEN, rect)
def eat(self, food):
if self.body[] == food:
self.body.append(self.body[-1])
def collide(self):
head = self.body[]
if head[] < or head[] >= WINDOW_WIDTH // BLOCK_SIZE:
return True
if head[1] < or head[1] >= WINDOW_HEIGHT // BLOCK_SIZE:
return True
for block in self.body[1:]:
if head == block:
return True
return False
# 定义食物类
class Food:
def __init__(self):
self.position = (random.randint(, WINDOW_WIDTH // BLOCK_SIZE - 1), random.randint(, WINDOW_HEIGHT // BLOCK_SIZE - 1))
def draw(self):
rect = pygame.Rect(self.position[] * BLOCK_SIZE, self.position[1] * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
pygame.draw.rect(window, RED, rect)
# 创建贪吃蛇和食物对象
snake = Snake()
food = Food()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT and snake.direction != 'left':
snake.direction = 'right'
elif event.key == pygame.K_LEFT and snake.direction != 'right':
snake.direction = 'left'
elif event.key == pygame.K_UP and snake.direction != 'down':
snake.direction = 'up'
elif event.key == pygame.K_DOWN and snake.direction != 'up':
snake.direction = 'down'
# 移动贪吃蛇
snake.move()
# 判断是否吃到食物
snake.eat(food.position)
# 判断是否碰撞到边界或自身
if snake.collide():
pygame.quit()
exit()
# 绘制游戏界面
window.fill(BLACK)
snake.draw()
food.draw()
pygame.display.update()
# 控制游戏帧率
clock.tick(10)
```
希望这个代码示例能够帮助您编写贪吃蛇游戏。
阅读全文
相关推荐








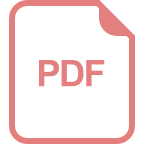
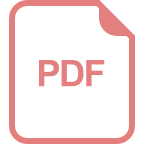
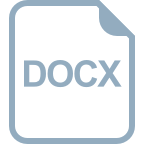