用python写出一个贪吃蛇游戏
时间: 2023-07-18 22:43:36 浏览: 83
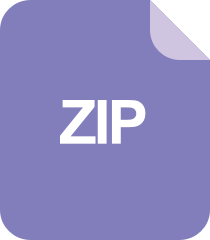
Python编写一个贪吃蛇游戏
好的,下面是一个简单的贪吃蛇游戏的 Python 代码实现,供参考:
```python
import pygame
import random
# 初始化 pygame
pygame.init()
# 游戏区域大小
width = 500
height = 500
# 颜色定义
white = (255, 255, 255)
red = (255, 0, 0)
black = (0, 0, 0)
# 创建游戏窗口
game_window = pygame.display.set_mode((width, height))
pygame.display.set_caption("贪吃蛇游戏")
# 蛇的坐标和方向
snake_x = 50
snake_y = 50
snake_size = 10
snake_list = []
snake_length = 1
snake_direction = "RIGHT"
# 食物的坐标
food_x = random.randint(0, width - 10)
food_y = random.randint(0, height - 10)
# 游戏结束标志
game_over = False
# 定义游戏时钟
clock = pygame.time.Clock()
# 定义字体
font = pygame.font.SysFont(None, 30)
# 显示得分函数
def display_score(score):
score_text = font.render("得分:" + str(score), True, black)
game_window.blit(score_text, [0, 0])
# 显示蛇的函数
def display_snake(snake_list):
for x,y in snake_list:
pygame.draw.rect(game_window, black, [x, y, snake_size, snake_size])
# 游戏主循环
while not game_over:
# 事件处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake_direction = "UP"
elif event.key == pygame.K_DOWN:
snake_direction = "DOWN"
elif event.key == pygame.K_LEFT:
snake_direction = "LEFT"
elif event.key == pygame.K_RIGHT:
snake_direction = "RIGHT"
# 移动蛇的位置
if snake_direction == "UP":
snake_y -= 10
elif snake_direction == "DOWN":
snake_y += 10
elif snake_direction == "LEFT":
snake_x -= 10
elif snake_direction == "RIGHT":
snake_x += 10
# 判断蛇是否吃到了食物
if abs(snake_x - food_x) < 10 and abs(snake_y - food_y) < 10:
food_x = random.randint(0, width - 10)
food_y = random.randint(0, height - 10)
snake_length += 1
# 更新蛇的列表
snake_head = []
snake_head.append(snake_x)
snake_head.append(snake_y)
snake_list.append(snake_head)
if len(snake_list) > snake_length:
del snake_list[0]
# 判断蛇是否撞墙或撞到自己
if snake_x < 0 or snake_x > width or snake_y < 0 or snake_y > height:
game_over = True
if snake_head in snake_list[:-1]:
game_over = True
# 绘制游戏窗口
game_window.fill(white)
pygame.draw.rect(game_window, red, [food_x, food_y, snake_size, snake_size])
display_snake(snake_list)
display_score(snake_length - 1)
pygame.display.update()
# 控制游戏帧率
clock.tick(15)
# 退出游戏
pygame.quit()
quit()
```
这是一个简单的实现,你可以根据自己的需求和想法进行修改和扩展。
阅读全文
相关推荐
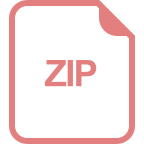












