python 用type()函数分别对整数 浮点数 字符串进行类型判断
时间: 2024-03-20 11:44:16 浏览: 20
当我们使用 type() 函数时,它会返回给我们所要判断的对象的类型。
以下是分别对整数、浮点数和字符串进行类型判断的 Python 代码:
```python
# 整数类型判断
num = 10
print(type(num))
# 浮点数类型判断
num_float = 3.14
print(type(num_float))
# 字符串类型判断
str_var = "Hello, world!"
print(type(str_var))
```
运行上述代码,输出结果如下:
```
<class 'int'>
<class 'float'>
<class 'str'>
```
可以看到,type() 函数分别返回了整数类型 int、浮点数类型 float 和字符串类型 str。
相关问题
请使用type()函数分别对整数,浮点数,字符串进行类型判断。python
好的!以下是对整数、浮点数、字符串分别进行类型判断的代码:
```python
# 整数类型判断
num1 = 10
print(type(num1)) # 输出 <class 'int'>
# 浮点数类型判断
num2 = 3.14
print(type(num2)) # 输出 <class 'float'>
# 字符串类型判断
str1 = "Hello, world!"
print(type(str1)) # 输出 <class 'str'>
```
以上代码中,`type()`函数可以返回其参数的数据类型。对于整数、浮点数和字符串,分别输出了它们的类型:`<class 'int'>`、`<class 'float'>`和`<class 'str'>`。
写出一段python代码 请使用type()函数分别对整数、浮点数、字符串进行类型判断。
以下是代码示例:
```python
x = 123 # 定义整数 x
y = 3.14 # 定义浮点数 y
z = "Hello, World!" # 定义字符串 z
print("x 的类型是:", type(x))
print("y 的类型是:", type(y))
print("z 的类型是:", type(z))
```
输出结果如下:
```
x 的类型是: <class 'int'>
y 的类型是: <class 'float'>
z 的类型是: <class 'str'>
```
解释:
- 使用 type 函数可以返回一个对象的类型。
- 对于整数和浮点数,它们的类型分别为 int 和 float。
- 对于字符串,它的类型为 str。
相关推荐
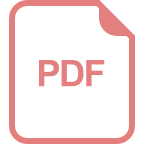
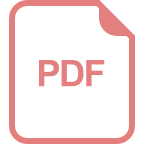
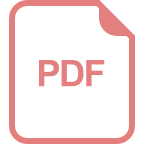












