禁忌搜索算法0/1背包问题 python实现思路
时间: 2023-10-22 09:36:17 浏览: 191
禁忌搜索算法是一种优化算法,用于解决NP难问题,如0/1背包问题。下面是禁忌搜索算法0/1背包问题的Python实现思路:
1. 随机生成一个初始解,即随机选择一些物品放入背包中。
2. 根据初始解,计算出初始解的价值。
3. 设定一个禁忌表,用于记录已经搜索过的解,以避免重复搜索。
4. 对于每个搜索步骤,根据当前解,生成一组邻居解。邻居解可以通过交换两个物品的位置或添加或删除一个物品来得到。
5. 对于每个邻居解,计算其价值,并判断其是否在禁忌表中。如果未在禁忌表中,则将其加入候选解集合中。
6. 从候选解集合中选择一个最优解作为下一次搜索的解,并将其加入禁忌表中。
7. 重复步骤4-6,直到达到停止条件。
8. 返回最优解。
下面是一个示例代码:
```python
import random
# 物品重量和价值
weights = [2, 3, 4, 5, 9]
values = [3, 4, 5, 8, 10]
# 背包容量和禁忌表大小
capacity = 20
tabu_size = 5
# 生成初始解
def generate_initial_solution():
solution = []
while sum(solution) < capacity:
item = random.randint(0, len(weights) - 1)
if weights[item] + sum(solution) <= capacity:
solution.append(item)
return solution
# 计算解的价值
def evaluate_solution(solution):
value = 0
for item in solution:
value += values[item]
return value
# 生成邻居解
def generate_neighbors(solution):
neighbors = []
for i in range(len(solution)):
for j in range(i + 1, len(solution)):
neighbor = solution.copy()
neighbor[i], neighbor[j] = neighbor[j], neighbor[i]
neighbors.append(neighbor)
for i in range(len(solution)):
neighbor = solution.copy()
neighbor.pop(i)
neighbors.append(neighbor)
for j in range(len(weights)):
if j not in neighbor and weights[j] + sum(neighbor) <= capacity:
neighbor.append(j)
neighbors.append(neighbor)
return neighbors
# 禁忌搜索算法
def taboo_search():
tabu_list = []
current_solution = generate_initial_solution()
current_value = evaluate_solution(current_solution)
best_solution = current_solution
best_value = current_value
while len(tabu_list) < tabu_size:
tabu_list.append(current_solution)
while True:
neighbors = generate_neighbors(current_solution)
candidate_solutions = []
for neighbor in neighbors:
if neighbor not in tabu_list:
candidate_solutions.append(neighbor)
if not candidate_solutions:
break
candidate_values = [evaluate_solution(solution) for solution in candidate_solutions]
best_candidate_value = max(candidate_values)
best_candidate_index = candidate_values.index(best_candidate_value)
best_candidate_solution = candidate_solutions[best_candidate_index]
if best_candidate_value > best_value:
best_solution = best_candidate_solution
best_value = best_candidate_value
current_solution = best_candidate_solution
current_value = best_candidate_value
tabu_list.pop(0)
tabu_list.append(current_solution)
return best_solution, best_value
# 测试
best_solution, best_value = taboo_search()
print('最优解:', best_solution)
print('最优解的价值:', best_value)
```
这段代码实现了禁忌搜索算法解决0/1背包问题的过程。在代码中,我们先定义了物品的重量和价值,背包的容量以及禁忌表的大小。然后,我们生成初始解并计算其价值。接着,我们在循环中不断生成邻居解,并计算其价值。如果邻居解不在禁忌表中,则将其加入候选解集合中。从候选解集合中选择一个最优解作为下一次搜索的解,并将其加入禁忌表中。最后,返回最优解。
阅读全文
相关推荐




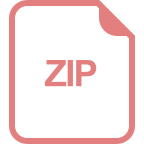









