禁忌搜索算法背包问题python
时间: 2023-06-22 21:46:06 浏览: 152
禁忌搜索算法是一种启发式搜索算法,用于解决组合优化问题,例如背包问题。下面是一个使用禁忌搜索算法解决背包问题的Python实现:
```python
import random
def knapsack(items, capacity, tabu_size, max_iter):
# 初始化禁忌表和当前解
tabu_list = []
current_solution = [0] * len(items)
current_weight = 0
current_value = 0
best_solution = current_solution
best_value = 0
# 迭代
for i in range(max_iter):
# 生成邻域解
neighbors = []
for j in range(len(items)):
neighbor = current_solution.copy()
neighbor[j] = 1 - neighbor[j]
if neighbor not in tabu_list:
neighbors.append(neighbor)
# 选择最优邻域解
best_neighbor = None
best_neighbor_value = 0
for neighbor in neighbors:
neighbor_weight = sum([item[0] for item in items if neighbor[items.index(item)]])
if neighbor_weight <= capacity:
neighbor_value = sum([item[1] for item in items if neighbor[items.index(item)]])
if neighbor_value > best_neighbor_value:
best_neighbor = neighbor
best_neighbor_value = neighbor_value
# 更新当前解和最优解
if best_neighbor:
current_solution = best_neighbor
current_weight = sum([item[0] for item in items if current_solution[items.index(item)]])
current_value = sum([item[1] for item in items if current_solution[items.index(item)]])
if current_value > best_value:
best_solution = current_solution
best_value = current_value
# 更新禁忌表
if len(tabu_list) >= tabu_size:
tabu_list.pop(0)
tabu_list.append(current_solution)
return best_solution, best_value
```
该函数接受三个参数:物品列表,背包容量,禁忌表大小和最大迭代次数。其中,物品列表包含每个物品的重量和价值,如[(4, 8), (3, 6), (1, 2), (5, 10)]表示有4个物品,它们的重量和价值分别为(4, 8), (3, 6), (1, 2), (5, 10)。禁忌表用于记录已经搜索过的解,避免重复搜索。在每次迭代中,算法会生成邻域解并选择最优邻域解更新当前解和最优解。如果当前解比最优解更好,则将当前解作为新的最优解。如果当前解是一个禁忌解,则不会更新禁忌表,否则将当前解添加到禁忌表中。最终,函数返回最优解和最优解的价值。
阅读全文
相关推荐
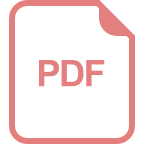


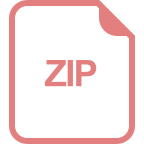
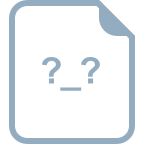
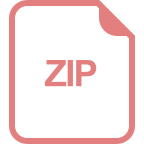
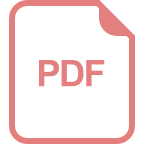
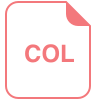
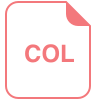
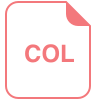
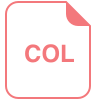
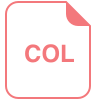
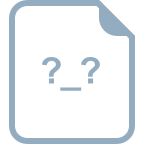