描述vue3页面的路由配置、组件拆分
时间: 2023-10-08 14:04:34 浏览: 164
好的,我来为您描述一下 Vue3 页面的路由配置和组件拆分。
## 路由配置
Vue3 的路由使用 Vue Router 4,其路由配置的基本步骤如下:
1. 首先需要安装 Vue Router 4,可以通过 npm 或者 yarn 进行安装。
```
npm install vue-router@4
```
2. 在 main.js 中引入 Vue Router,并使用 `createRouter` 创建一个路由实例。
```javascript
import { createApp } from 'vue'
import App from './App.vue'
import { createRouter, createWebHistory } from 'vue-router'
import Home from './components/Home.vue'
import About from './components/About.vue'
const router = createRouter({
history: createWebHistory(),
routes: [
{ path: '/', component: Home },
{ path: '/about', component: About }
]
})
const app = createApp(App)
app.use(router)
app.mount('#app')
```
3. 在组件中使用 `<router-view>` 标签来展示路由组件。
```html
<template>
<div>
<h1>Home Page</h1>
</div>
</template>
```
## 组件拆分
Vue3 推荐使用组合式 API 进行组件拆分,可以将相关的逻辑和代码放在一个函数中,提高组件的可维护性和可读性。
例如,我们可以将一个组件的逻辑拆分成以下几个部分:
1. `setup` 部分:定义组件的数据和方法。
```javascript
import { ref } from 'vue'
export default {
setup() {
const count = ref(0)
const increment = () => {
count.value++
}
return {
count,
increment
}
}
}
```
2. `template` 部分:定义组件的 HTML 模板。
```html
<template>
<div>
<h1>Counter: {{ count }}</h1>
<button @click="increment">Increment</button>
</div>
</template>
```
3. `style` 部分:定义组件的 CSS 样式。
```css
<style scoped>
h1 {
color: red;
}
</style>
```
通过以上方式可以将一个组件进行拆分,提高组件的可维护性和可读性。
阅读全文
相关推荐
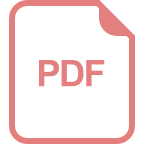
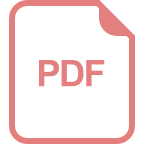
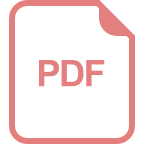
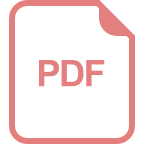


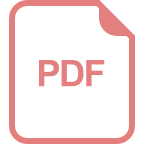
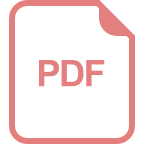
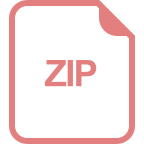
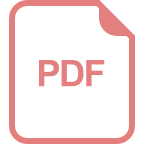
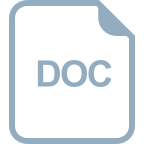
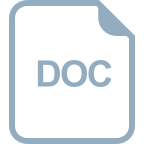
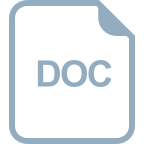
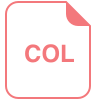
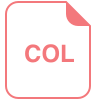
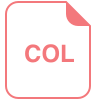
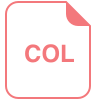

