class MyDataset(Dataset): def __init__(self, num_samples): super(MyDataset, self).__init__() self.num_samples = num_samples # 在 `__init__` 中定义数据增强方法,此处为调整图像大小 self.transform = Compose([Resize(size=32)])
时间: 2024-04-02 11:36:04 浏览: 77
这段代码定义了一个自定义数据集`MyDataset`,继承自`paddle.io.Dataset`。`num_samples`表示数据集中的样本数量。在`__init__`方法中,通过调用父类的`__init__`方法初始化实例,并将`num_samples`赋值给实例变量`self.num_samples`。此外,`transform`变量定义了对数据集进行的数据增强方法,其中`Compose`为将多个数据增强方法组合起来,`Resize(size=32)`定义了将数据集中的图片大小调整为32x32。该自定义数据集可以用于训练深度学习模型。
相关问题
import jieba from collections import Counter def read_dataset(path): labels = [] inputs = [] with open(path, 'r', encoding='utf-8') as file: for i, line in enumerate(file): line = line.strip() sample = line.split('\t') inputs.append(sample[0]) labels.append(sample[1]) return inputs, labels class MyDataset(): def init(self) -> None: self.vocab = {} self.stop_words = [] def set_stopword(self, path='data/scu_stopwords'): with open(path, 'r', encoding='utf-8') as fr: self.stop_words = [line.strip() for line in fr.readline()] def build_vocab(self, inputs, max_size='5000', min_freg=1): cnt = {} # 临时词典存储词频 for data in inputs: data = jieba.lcut(data) for word in data: if word not in cnt: cnt[word] = 1 else: cnt[word] += 1 cnt = sorted([_ for _ in cnt.items() if _[1]>=min_freg and _[0] not in self.stop_words], key=lambda t:t[1], reverse=True) self.vocab['<pad>'] = 0 if len(cnt) > max_size: i = 1 for w, _ in cnt: if len(self.vocab)>max_size: break self.vocab[w] = i i += 1 else: i = 1 for w, _ in cnt: self.vocab[w] = i i += 1 def transform(self, inputs, flag = 0): samples = [] iter = 0 for doc in inputs: if iter % 1000 == 0: print('-------%d------' % iter) doc = jieba.cut(doc) if flag==0: wordset = set(doc) # print(wordset) sample = [] for word in self.vocab.keys(): if word in wordset: sample.append(1) else: sample.append(0) elif flag == 1: sample = [0 for i in range(len(self.vocab.items()))] word_count = Counter(doc) for word in word_count.items(): if word[0] in self.vocab.keys(): id = self.vocab[word[0]] sample[id] = word[1] iter +=1 samples.append(sample) return samples def buid_tfidf_vocab(self, inputs, max_size): pass试着调参重构,提升精确率
很抱歉,您的代码片段缺少模型和评估指标等关键信息,我无法直接提供调参建议。不过,一些常见的提升精确率的方法包括:
- 调整模型结构,可以尝试更深的网络层、增加隐藏单元、添加正则化等等;
- 调整超参数,如学习率、批量大小、迭代次数等;
- 数据增强,可以尝试增加数据量、添加噪声、旋转等等;
- 特征工程,例如添加关键词、文本长度、情感分析等特征;
- 集成方法,例如结合多个模型,使用投票或加权平均等方式提升精度。
当然,具体方法需要结合您的具体任务和数据集情况进行调参和优化。
File "/Users/liudianhewen/Desktop/气象局实践/ganomaly-master/lib/data.py", line 354, in load_data dataloader = {x: torch.utils.data.DataLoader(dataset=dataset[x], File "/Users/liudianhewen/Desktop/气象局实践/ganomaly-master/lib/data.py", line 354, in <dictcomp> dataloader = {x: torch.utils.data.DataLoader(dataset=dataset[x], File "/opt/anaconda3/envs/python3812/lib/python3.8/site-packages/torch/utils/data/dataloader.py", line 277, in __init__ sampler = RandomSampler(dataset, generator=generator) # type: ignore[arg-type] File "/opt/anaconda3/envs/python3812/lib/python3.8/site-packages/torch/utils/data/sampler.py", line 96, in __init__ if not isinstance(self.num_samples, int) or self.num_samples <= 0: File "/opt/anaconda3/envs/python3812/lib/python3.8/site-packages/torch/utils/data/sampler.py", line 104, in num_samples return len(self.data_source) TypeError: object of type 'Dataset' has no len()
根据错误信息来看,代码中存在一个 `TypeError` 错,错误信息显示为 `object of type 'Dataset' has no len()`。该错误发生在试图获取数据集的长度时出现问题。
这个错误通常是由于数据集对象(`Dataset`)没有实现 `len()` 方法导致的。在使用 `torch.utils.data.DataLoader` 创建数据加载器时,需要传入一个数据集对象,并且数据集对象需要实现 `len()` 方法来返回数据集的长度。
解决这个问题的方法是确保你使用的数据集对象具有 `len()` 方法。如果你使用的是 PyTorch 提供的内置数据集类(如 `torchvision.datasets.ImageFolder`),那么通常不会遇到这个问题。但如果你自定义了一个数据集类,你需要确保在该类中实现了 `len()` 方法。
以下是一个自定义数据集类的示例,展示了如何实现 `len()` 方法:
```python
import torch
from torch.utils.data import Dataset
class MyDataset(Dataset):
def __init__(self, data):
self.data = data
def __getitem__(self, index):
return self.data[index]
def __len__(self):
return len(self.data)
```
在上面的示例中,我们创建了一个名为 `MyDataset` 的自定义数据集类。它接受一个数据列表作为参数,并在 `__getitem__` 方法中实现了获取单个样本的逻辑。同时,我们在 `__len__` 方法中返回了数据列表的长度。这样,我们就可以在使用 `torch.utils.data.DataLoader` 创建数据加载器时,传入 `MyDataset` 对象,而不会再出现 `TypeError` 错误。
阅读全文
相关推荐
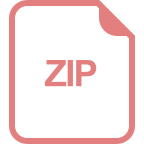
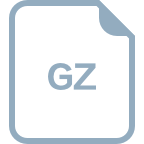
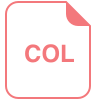
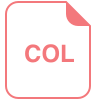




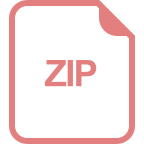