The readLine method uses System.out.print (not println) to print its string argument on the screen (later when we use the readLine method, the string argument of the method will be a message telling the user to type some text). Then the readLine method uses the input scanner object to read a whole line of text from the user of the program and returns the text as result. The readPosInt method uses System.out.print (not println) to print its string argument on the screen (later when we use the readPosInt method, the string argument of the method will be a message telling the user to type some integer). Then the readPosInt method uses the input scanner object to read an integer from the user of the program.写一个java文件
时间: 2023-12-14 14:38:48 浏览: 130
下面是一个实现了readLine和readPosInt方法的Java文件:
```java
import java.util.Scanner;
public class InputReader {
private Scanner input;
public InputReader() {
input = new Scanner(System.in);
}
public String readLine(String message) {
System.out.print(message);
return input.nextLine();
}
public int readPosInt(String message) {
System.out.print(message);
int num = input.nextInt();
input.nextLine(); // consume newline character
while (num <= 0) {
System.out.print("Please enter a positive integer: ");
num = input.nextInt();
input.nextLine(); // consume newline character
}
return num;
}
}
```
这个类有一个私有的Scanner对象,用于读取用户的输入。readLine方法和readPosInt方法都接受一个String类型的参数作为提示信息,然后通过System.out.print方法将提示信息打印到屏幕上。readLine方法调用input.nextLine()方法读取用户输入的一行文本,并返回该文本。readPosInt方法调用input.nextInt()方法读取用户输入的整数,然后消耗掉输入中的换行符(因为nextInt方法只会读取整数部分,而不包括后面的换行符)。然后,如果输入的整数不是正整数,就会提示用户重新输入,直到输入的整数为正整数为止。最后,readPosInt方法返回输入的正整数。
阅读全文
相关推荐
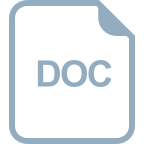
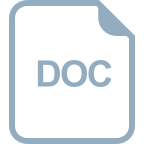
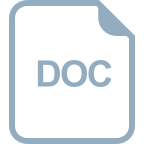

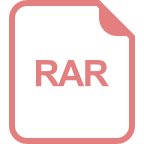
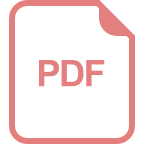






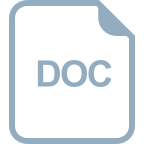
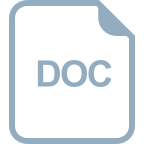
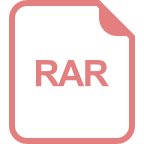
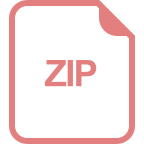