用python,html和MySQL,写一个物流运输管理系统,其中包括登录页面,订单管理,车辆调度,和车辆跟踪四个页面,订单管理和车辆调度以及车辆跟踪这三个页面,每个页面可以查询,添加和删除信息,并且可以显示在页面上
时间: 2023-10-05 09:14:39 浏览: 43
实现这个物流运输管理系统需要以下步骤:
1.设计数据库
首先需要设计一个数据库来存储订单和车辆信息。可以使用MySQL或者其他关系数据库来实现。设计好数据库表结构后,可以通过Python的MySQLdb模块来实现数据的增删改查操作。
2.设计登录页面
登录页面需要包括输入用户名和密码的表单,以及登录按钮。可以使用HTML和CSS来设计登录页面,使用Python和Flask框架来实现用户登录的认证和授权功能。
3.设计订单管理页面
订单管理页面需要显示所有订单的信息,并且可以查询、添加和删除订单。可以使用HTML和CSS来设计订单管理页面,使用Python和Flask框架来实现对订单的增删改查功能。
4.设计车辆调度页面
车辆调度页面需要显示所有车辆的信息,并且可以查询、添加和删除车辆。可以使用HTML和CSS来设计车辆调度页面,使用Python和Flask框架来实现对车辆的增删改查功能。
5.设计车辆跟踪页面
车辆跟踪页面需要显示所有车辆的实时位置,并且可以根据车辆编号查询车辆的位置信息。可以使用HTML和CSS来设计车辆跟踪页面,使用JavaScript和AJAX来实现实时更新车辆位置信息的功能。
6.实现整个系统
将以上设计好的页面和功能整合在一起,就可以实现一个完整的物流运输管理系统了。
示例代码如下:
(1)设计数据库
```sql
CREATE TABLE orders (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
address VARCHAR(100) NOT NULL,
phone VARCHAR(20) NOT NULL,
status ENUM('待发货', '已发货', '已签收') NOT NULL DEFAULT '待发货',
PRIMARY KEY (id)
);
CREATE TABLE vehicles (
id INT(11) NOT NULL AUTO_INCREMENT,
plate_number VARCHAR(20) NOT NULL,
driver_name VARCHAR(50) NOT NULL,
status ENUM('空闲', '运输中') NOT NULL DEFAULT '空闲',
PRIMARY KEY (id)
);
```
(2)设计登录页面
```html
<!DOCTYPE html>
<html>
<head>
<title>登录页面</title>
<link rel="stylesheet" type="text/css" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h2>请登录</h2>
<form method="POST" action="{{ url_for('login') }}">
<div>
<label>用户名:</label>
<input type="text" name="username">
</div>
<div>
<label>密码:</label>
<input type="password" name="password">
</div>
<div>
<input type="submit" value="登录">
</div>
</form>
</body>
</html>
```
```python
from flask import Flask, render_template, request, session, redirect, url_for
app = Flask(__name__)
app.secret_key = 'secret_key'
@app.route('/')
def index():
return render_template('login.html')
@app.route('/login', methods=['POST'])
def login():
username = request.form['username']
password = request.form['password']
if username == 'admin' and password == 'admin':
session['username'] = username
return redirect(url_for('orders'))
else:
return redirect(url_for('index'))
@app.route('/logout')
def logout():
session.pop('username', None)
return redirect(url_for('index'))
```
(3)设计订单管理页面
```html
<!DOCTYPE html>
<html>
<head>
<title>订单管理</title>
<link rel="stylesheet" type="text/css" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h2>订单管理</h2>
<div>
<form method="GET" action="{{ url_for('orders') }}">
<input type="text" name="search" placeholder="按姓名或地址搜索">
<input type="submit" value="搜索">
</form>
</div>
<div>
<form method="POST" action="{{ url_for('add_order') }}">
<div>
<label>姓名:</label>
<input type="text" name="name">
</div>
<div>
<label>地址:</label>
<input type="text" name="address">
</div>
<div>
<label>电话:</label>
<input type="text" name="phone">
</div>
<div>
<input type="submit" value="添加">
</div>
</form>
</div>
<table>
<tr>
<th>ID</th>
<th>姓名</th>
<th>地址</th>
<th>电话</th>
<th>状态</th>
<th>操作</th>
</tr>
{% for order in orders %}
<tr>
<td>{{ order.id }}</td>
<td>{{ order.name }}</td>
<td>{{ order.address }}</td>
<td>{{ order.phone }}</td>
<td>{{ order.status }}</td>
<td>
<form method="POST" action="{{ url_for('delete_order', id=order.id) }}">
<input type="submit" value="删除">
</form>
</td>
</tr>
{% endfor %}
</table>
</body>
</html>
```
```python
import MySQLdb
@app.route('/orders')
def orders():
if 'username' not in session:
return redirect(url_for('index'))
conn = MySQLdb.connect(host='localhost', user='root', password='password', db='database')
cursor = conn.cursor()
if 'search' in request.args:
search = request.args['search']
cursor.execute("SELECT * FROM orders WHERE name LIKE %s OR address LIKE %s", ('%' + search + '%', '%' + search + '%'))
else:
cursor.execute("SELECT * FROM orders")
orders = []
for row in cursor.fetchall():
order = {}
order['id'] = row[0]
order['name'] = row[1]
order['address'] = row[2]
order['phone'] = row[3]
order['status'] = row[4]
orders.append(order)
conn.close()
return render_template('orders.html', orders=orders)
@app.route('/add_order', methods=['POST'])
def add_order():
if 'username' not in session:
return redirect(url_for('index'))
conn = MySQLdb.connect(host='localhost', user='root', password='password', db='database')
cursor = conn.cursor()
name = request.form['name']
address = request.form['address']
phone = request.form['phone']
cursor.execute("INSERT INTO orders (name, address, phone) VALUES (%s, %s, %s)", (name, address, phone))
conn.commit()
conn.close()
return redirect(url_for('orders'))
@app.route('/delete_order/<int:id>', methods=['POST'])
def delete_order(id):
if 'username' not in session:
return redirect(url_for('index'))
conn = MySQLdb.connect(host='localhost', user='root', password='password', db='database')
cursor = conn.cursor()
cursor.execute("DELETE FROM orders WHERE id = %s", (id,))
conn.commit()
conn.close()
return redirect(url_for('orders'))
```
(4)设计车辆调度页面
```html
<!DOCTYPE html>
<html>
<head>
<title>车辆调度</title>
<link rel="stylesheet" type="text/css" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h2>车辆调度</h2>
<div>
<form method="GET" action="{{ url_for('vehicles') }}">
<input type="text" name="search" placeholder="按车牌号或司机姓名搜索">
<input type="submit" value="搜索">
</form>
</div>
<div>
<form method="POST" action="{{ url_for('add_vehicle') }}">
<div>
<label>车牌号:</label>
<input type="text" name="plate_number">
</div>
<div>
<label>司机姓名:</label>
<input type="text" name="driver_name">
</div>
<div>
<input type="submit" value="添加">
</div>
</form>
</div>
<table>
<tr>
<th>ID</th>
<th>车牌号</th>
<th>司机姓名</th>
<th>状态</th>
<th>操作</th>
</tr>
{% for vehicle in vehicles %}
<tr>
<td>{{ vehicle.id }}</td>
<td>{{ vehicle.plate_number }}</td>
<td>{{ vehicle.driver_name }}</td>
<td>{{ vehicle.status }}</td>
<td>
<form method="POST" action="{{ url_for('delete_vehicle', id=vehicle.id) }}">
<input type="submit" value="删除">
</form>
</td>
</tr>
{% endfor %}
</table>
</body>
</html>
```
```python
@app.route('/vehicles')
def vehicles():
if 'username' not in session:
return redirect(url_for('index'))
conn = MySQLdb.connect(host='localhost', user='root', password='password', db='database')
cursor = conn.cursor()
if 'search' in request.args:
search = request.args['search']
cursor.execute("SELECT * FROM vehicles WHERE plate_number LIKE %s OR driver_name LIKE %s", ('%' + search + '%', '%' + search + '%'))
else:
cursor.execute("SELECT * FROM vehicles")
vehicles = []
for row in cursor.fetchall():
vehicle = {}
vehicle['id'] = row[0]
vehicle['plate_number'] = row[1]
vehicle['driver_name'] = row[2]
vehicle['status'] = row[3]
vehicles.append(vehicle)
conn.close()
return render_template('vehicles.html', vehicles=vehicles)
@app.route('/add_vehicle', methods=['POST'])
def add_vehicle():
if 'username' not in session:
return redirect(url_for('index'))
conn = MySQLdb.connect(host='localhost', user='root', password='password', db='database')
cursor = conn.cursor()
plate_number = request.form['plate_number']
driver_name = request.form['driver_name']
cursor.execute("INSERT INTO vehicles (plate_number, driver_name) VALUES (%s, %s)", (plate_number, driver_name))
conn.commit()
conn.close()
return redirect(url_for('vehicles'))
@app.route('/delete_vehicle/<int:id>', methods=['POST'])
def delete_vehicle(id):
if 'username' not in session:
return redirect(url_for('index'))
conn = MySQLdb.connect(host='localhost', user='root', password='password', db='database')
cursor = conn.cursor()
cursor.execute("DELETE FROM vehicles WHERE id = %s", (id,))
conn.commit()
conn.close()
return redirect(url_for('vehicles'))
```
(5)设计车辆跟踪页面
```html
<!DOCTYPE html>
<html>
<head>
<title>车辆跟踪</title>
<meta http-equiv="refresh" content="10">
<link rel="stylesheet" type="text/css" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h2>车辆跟踪</h2>
<div>
<form method="GET" action="{{ url_for('track') }}">
<input type="text" name="plate_number" placeholder="请输入车牌号">
<input type="submit" value="查找">
</form>
</div>
{% if vehicle is not none %}
<div>
<h3>车牌号:{{ vehicle.plate_number }}</h3>
<h3>司机姓名:{{ vehicle.driver_name }}</h3>
<h3>状态:{{ vehicle.status }}</h3>
{% if location is not none %}
<h3>位置:{{ location.latitude }}, {{ location.longitude }}</h3>
{% endif %}
</div>
{% endif %}
<script src="{{ url_for('static', filename='jquery.min.js') }}"></script>
<script>
$(function() {
setInterval(function() {
$.get('{{ url_for('location', plate_number=vehicle.plate_number) }}', function(data) {
if (data) {
$('h3:contains("位置:")').text('位置:' + data.latitude + ', ' + data.longitude);
}
});
}, 10000);
});
</script>
</body>
</html>
```
```python
import requests, json
@app.route('/track')
def track():
if 'username' not in session:
return redirect(url_for('index'))
vehicle = None
location = None
if 'plate_number' in request.args:
plate_number = request.args['plate_number']
conn = MySQLdb.connect(host='localhost', user='root', password='password', db='database')
cursor = conn.cursor()
cursor.execute("SELECT * FROM vehicles WHERE plate_number = %s", (plate_number,))
row = cursor.fetchone()
if row is not None:
vehicle = {}
vehicle['id'] = row[0]
vehicle['plate_number'] = row[1]
vehicle['driver_name'] = row[2]
vehicle['status'] = row[3]
cursor.execute("SELECT * FROM locations WHERE vehicle_id = %s ORDER BY id DESC LIMIT 1", (row[0],))
row = cursor.fetchone()
if row is not None:
location = {}
location['id'] = row[0]
location['vehicle_id'] = row[1]
location['latitude'] = row[2]
location['longitude'] = row[3]
conn.close()
return render_template('track.html', vehicle=vehicle, location=location)
@app.route('/location/<string:plate_number>')
def location(plate_number):
conn = MySQLdb.connect(host='localhost', user='root', password='password', db='database')
cursor = conn.cursor()
cursor.execute("SELECT * FROM vehicles WHERE plate_number = %s", (plate_number,))
row = cursor.fetchone()
if row is not None:
cursor.execute("SELECT * FROM locations WHERE vehicle_id = %s ORDER BY id DESC LIMIT 1", (row[0],))
row = cursor.fetchone()
if row is not None:
location = {}
location['id'] = row[0]
location['vehicle_id'] = row[1]
location['latitude'] = row[2]
location['longitude'] = row[3]
return json.dumps(location)
conn.close()
return ''
```
(6)实现整个系统
在Python的Flask框架中,可以使用Blueprint来实现模块化的设计。将上述设计好的页面和功能按照模块划分,将其分别放在不同的Blueprint中,最后将它们注册到主应用程序中,就可以实现一个完整的物流运输管理系统了。
相关推荐
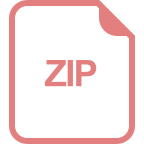
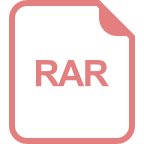
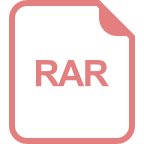
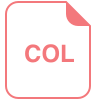
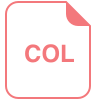
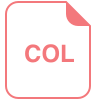
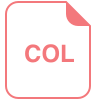
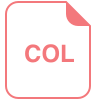










