registerButton.addEventListener('click', function(event) { event.preventDefault(); const username = registerUsernameInput.value; const email = registerEmailInput.value; const password = registerPasswordInput.value; const confirmPassword = registerConfirmPasswordInput.value;
时间: 2024-03-23 15:37:32 浏览: 16
这段代码是为注册按钮 `registerButton` 添加了一个点击事件的监听器。当用户点击注册按钮时,会执行回调函数。回调函数中的第一条语句 `event.preventDefault()` 是用来阻止表单默认的提交行为。这是因为表单默认的提交行为会导致页面刷新,而我们希望在不刷新页面的情况下通过 AJAX 提交表单。
接下来,代码从表单中获取了用户名、电子邮箱、密码和确认密码等信息,并将其保存在对应的变量中:
```javascript
const username = registerUsernameInput.value;
const email = registerEmailInput.value;
const password = registerPasswordInput.value;
const confirmPassword = registerConfirmPasswordInput.value;
```
其中,`registerUsernameInput`、`registerEmailInput`、`registerPasswordInput` 和 `registerConfirmPasswordInput` 是表单中对应的输入框元素。这些元素通常可以通过 `document.getElementById` 或 `document.querySelector` 方法来获取。
接下来的代码就是通过 AJAX 发送注册请求。具体实现可能会有所不同,但一般来说,会使用 `XMLHttpRequest` 对象或者 `fetch` API 来发送请求,并将表单数据作为请求体发送给服务器。
相关推荐
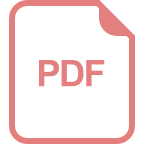














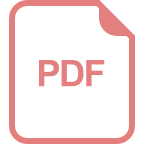