unity 按C键保存对象内rect Transform数据并生成一个txt,当程序重新运行读取数据并赋值到对象的rect Transform
时间: 2024-09-30 15:06:23 浏览: 115
在Unity中,要实现按C键保存Rect Transform数据并将其写入文本文件,你可以创建一个脚本,利用Unity内置的一些功能和C#编程来完成这个任务。以下是一个简单的步骤和代码示例:
1. 首先,在Unity编辑器里创建一个新的C#脚本(例如SaveRectTransform.cs),然后添加以下内容:
```csharp
using UnityEngine;
using System.IO;
public class SaveRectTransform : MonoBehaviour
{
public RectTransform rectTransformToSave; // 存储要保存的RectTransform
void OnEnable()
{
if (Input.GetKeyDown(KeyCode.C))
{
SaveRectData();
}
}
private void SaveRectData()
{
string filePath = Application.persistentDataPath + "/RectTransform.txt";
// 获取RectTransform的数据并序列化为字符串
Rect rect = rectTransformToSave.rect;
Vector3 pos = rectTransformToSave.position;
Quaternion rot = rectTransformToSave.rotation;
string data = $"Position: ({pos.x}, {pos.y}, {pos.z}),\nRotation: ({rot.x}, {rot.y}, {rot.z}),\nSize: ({rect.width}, {rect.height})";
try
{
using (StreamWriter writer = new StreamWriter(filePath))
{
writer.WriteLine(data);
Debug.Log("Rect Transform data saved to " + filePath);
}
}
catch (Exception ex)
{
Debug.LogError("Failed to save data: " + ex.Message);
}
}
// 当重新加载时,可以在这里恢复数据
void OnApplicationQuit()
{
LoadRectData();
}
private void LoadRectData()
{
string filePath = Application.persistentDataPath + "/RectTransform.txt";
if (File.Exists(filePath))
{
try
{
using (StreamReader reader = new StreamReader(filePath))
{
string[] lines = reader.ReadToEnd().Split('\n');
float[] positionValues = lines[0].Substring(9).Split(',').Select(float.Parse).ToArray();
float[] rotationValues = lines.Substring(11).Split(',').Select(float.Parse).ToArray();
float[] sizeValues = lines[2].Substring(7).Split(',').Select(float.Parse).ToArray();
rectTransformToSave.position = new Vector3(positionValues, positionValues, positionValues);
rectTransformToSave.rotation = Quaternion.Euler(rotationValues);
rectTransformToSave.sizeDelta = new Vector2(sizeValues, sizeValues);
Debug.Log("Rect Transform data loaded from " + filePath);
}
}
catch (Exception ex)
{
Debug.LogError("Failed to load data: " + ex.Message);
}
}
}
}
```
2. 将此脚本附加到一个GameObject上,确保`rectTransformToSave`组件引用了你想要保存的RectTransform。
当你按下C键时,`SaveRectData()`方法会被调用,会将RectTransform的位置、旋转和平移信息写入文件。如果游戏关闭再打开,`LoadRectData()`会在`OnApplicationQuit()`事件中执行,尝试从文件中读取数据并设置给Rect Transform。
阅读全文
相关推荐
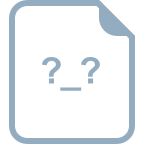
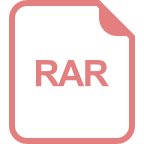




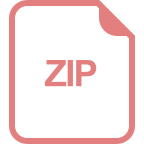
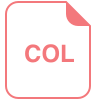
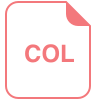



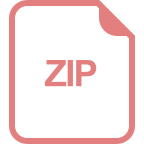