写一个在已有模型基础上,将图像分类的界面设计代码python
时间: 2023-06-04 11:06:35 浏览: 171
很高兴能够为您提供帮助,我将尝试回答您的问题。
以下是一些基于已有模型实现图像分类界面设计的Python代码:
```python
# 导入所需库
import tkinter as tk
from tkinter import filedialog
from PIL import ImageTk, Image
import numpy as np
import tensorflow as tf
# 加载模型
model = tf.keras.models.load_model('model.h5')
# 定义标签类别
class_labels = ['cat', 'dog']
# 创建图像分类应用程序
class ImageClassificationApp:
def __init__(self, master):
self.master = master
master.title('图像分类')
# 创建图像显示区域
self.image_label = tk.Label(master)
self.image_label.pack()
# 创建按钮
self.button = tk.Button(master, text='选择图像', command=self.choose_image)
self.button.pack()
# 创建标签区域
self.label = tk.Label(master, text='')
self.label.pack()
# 定义选择图像函数
def choose_image(self):
# 打开文件对话框
file_path = filedialog.askopenfilename(initialdir='/', title='选择图像文件',
filetypes=(('jpeg files', '*.jpg'), ('all files', '*.*')))
# 如果成功选择了文件
if file_path:
# 加载图像
image = Image.open(file_path)
# 将图像缩放到网络所需的大小
image = image.resize((150, 150))
# 将图像转化为灰度图像
image = image.convert('L')
# 将图像转化为numpy数组
image_array = np.array(image)
# 将图像数组标准化
image_array = image_array / 255.0
# 将图像数组增加一个维度
image_array = np.expand_dims(image_array, axis=0)
image_array = np.expand_dims(image_array, axis=3)
# 预测标签
predictions = model.predict(image_array)
classification = class_labels[np.argmax(predictions)]
# 更新图像和标签
self.update_image(image)
self.update_label(classification)
# 定义更新图像函数
def update_image(self, image):
self.image = ImageTk.PhotoImage(image)
self.image_label.configure(image=self.image)
# 定义更新标签函数
def update_label(self, classification):
self.label.configure(text='这是一个 ' + classification)
# 启动应用程序
root = tk.Tk()
app = ImageClassificationApp(root)
root.mainloop()
```
这段代码实现了基于已有模型的图像分类界面设计,在应用程序中用户可以选择一张图像并对其进行分类。如果您需要进一步的帮助,请告诉我。
阅读全文
相关推荐
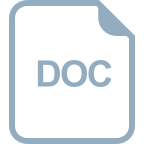
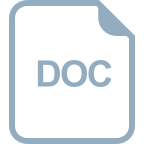
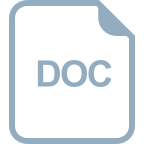
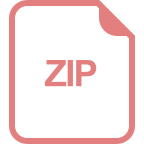
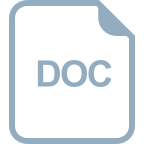
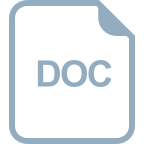
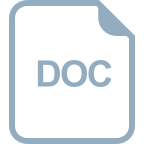
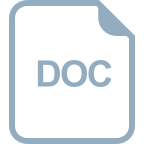
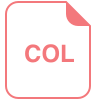







