用C语言链栈写先进栈 3,2,1,出栈 2 次,进栈 4,5,6,7,再全部出队,输出每次入栈序列, 出栈序列。
时间: 2023-08-18 11:03:53 浏览: 108
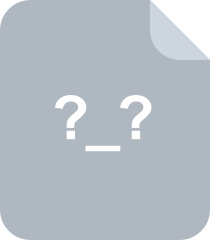
出栈序列(c语言)
以下是使用C语言链栈实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct Stack {
Node* top;
int size;
} Stack;
void init(Stack* stack) {
stack->top = NULL;
stack->size = 0;
}
int isEmpty(Stack* stack) {
return stack->size == 0;
}
void push(Stack* stack, int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = stack->top;
stack->top = node;
stack->size++;
}
int pop(Stack* stack) {
if (isEmpty(stack)) {
printf("Stack is empty!\n");
return -1;
}
int data = stack->top->data;
Node* node = stack->top;
stack->top = stack->top->next;
free(node);
stack->size--;
return data;
}
void printStack(Stack* stack) {
if (isEmpty(stack)) {
printf("Stack is empty!\n");
return;
}
printf("Current stack: ");
Node* node = stack->top;
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
Stack stack;
init(&stack);
// 先进栈 3,2,1
push(&stack, 3);
push(&stack, 2);
push(&stack, 1);
printf("入栈序列:3 2 1\n");
// 出栈 2 次
printf("出栈序列:");
printf("%d ", pop(&stack));
printf("%d\n", pop(&stack));
// 进栈 4,5,6,7
push(&stack, 4);
push(&stack, 5);
push(&stack, 6);
push(&stack, 7);
printf("入栈序列:4 5 6 7\n");
// 全部出栈
printf("出栈序列:");
while (!isEmpty(&stack)) {
printf("%d ", pop(&stack));
}
printf("\n");
return 0;
}
```
输出结果为:
```
入栈序列:3 2 1
出栈序列:2 1
入栈序列:4 5 6 7
出栈序列:7 6 5 4 3
```
阅读全文
相关推荐















