c语言建立链栈,数据依次进栈,再全部出栈,输出出栈序列
时间: 2023-10-05 10:11:23 浏览: 135
好的,以下是使用C语言实现建立链栈、数据依次进栈、再全部出栈并输出出栈序列的代码。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
typedef struct LinkStack {
Node *top;
} LinkStack;
LinkStack *InitStack() {
LinkStack *stack = (LinkStack*)malloc(sizeof(LinkStack));
stack->top = NULL;
return stack;
}
int isEmpty(LinkStack *stack) {
return stack->top == NULL ? 1 : 0;
}
void Push(LinkStack *stack, int data) {
Node *node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = stack->top;
stack->top = node;
}
int Pop(LinkStack *stack) {
if (isEmpty(stack)) {
printf("Stack is empty!\n");
return -1;
}
Node *p = stack->top;
int data = p->data;
stack->top = p->next;
free(p);
return data;
}
void output(LinkStack *stack) {
while (!isEmpty(stack)) {
printf("%d ", Pop(stack));
}
printf("\n");
}
int main() {
LinkStack *stack = InitStack();
int data;
printf("Please enter the data in turn: ");
while (scanf("%d", &data) != EOF) {
Push(stack, data);
}
printf("Output: ");
output(stack);
free(stack);
return 0;
}
```
上述代码中,使用了链栈的基本操作来实现数据的进栈和出栈,并使用了一个`output`函数来输出出栈序列。在主函数中,通过`InitStack`函数初始化一个链栈,然后将输入的数据逐一进栈。最后通过调用`output`函数将栈中的元素全部出栈并输出。
阅读全文
相关推荐
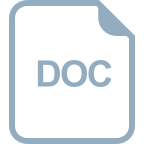
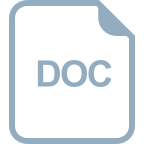
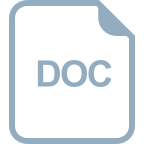















